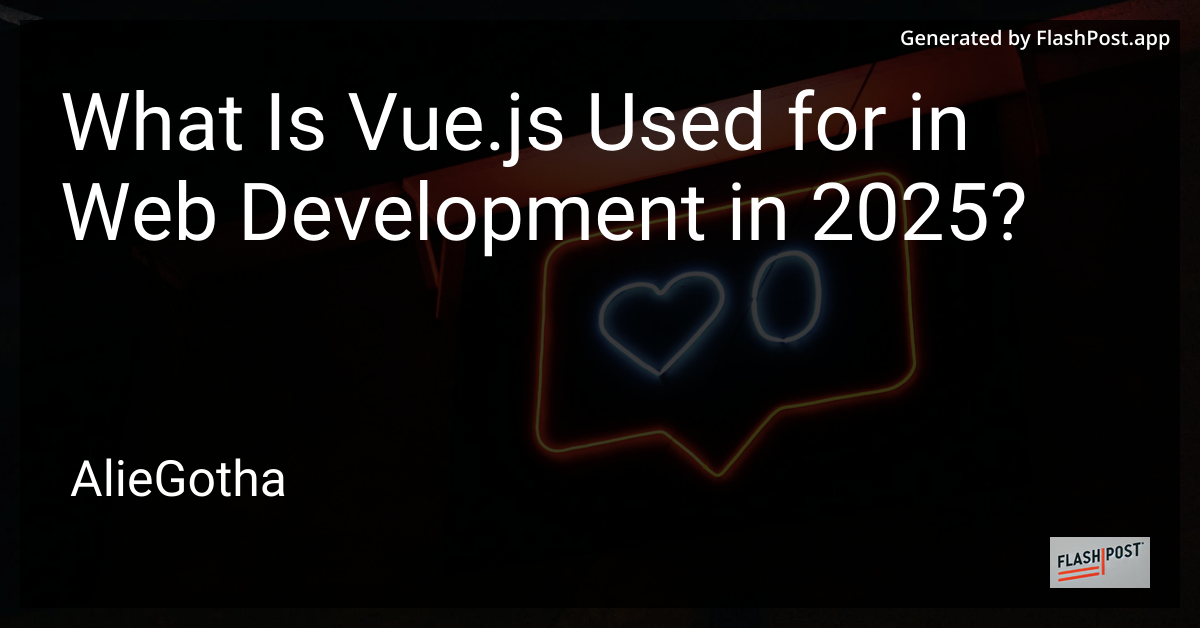
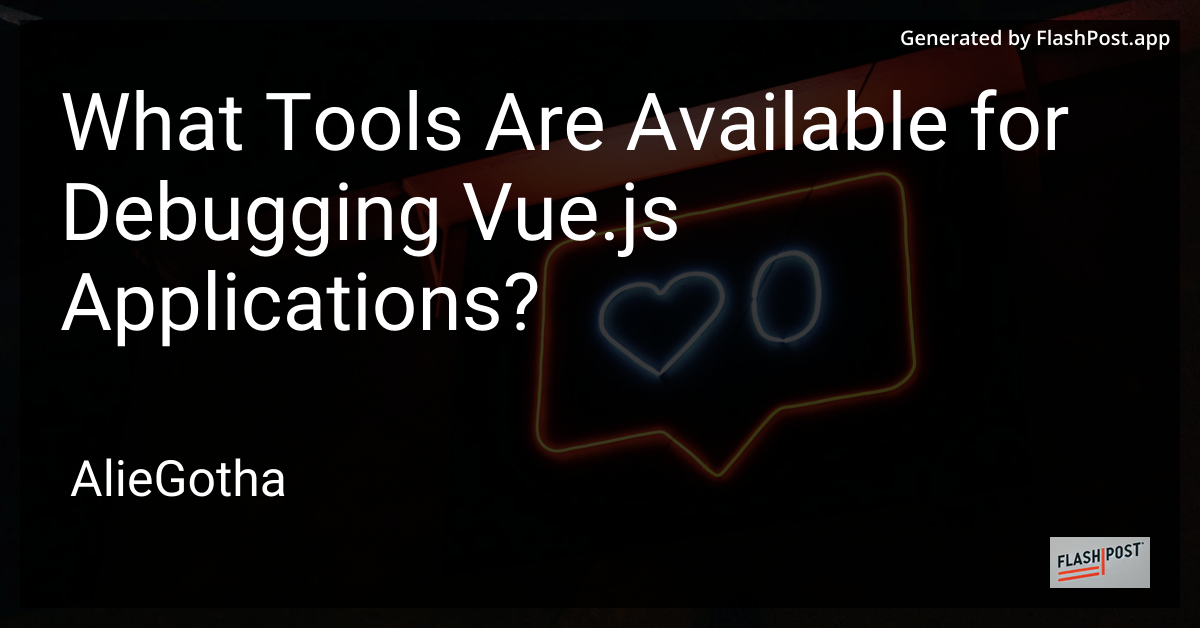
What Tools Are Available for Debugging Vue.js Applications?
Debugging is an integral part of the software development process, especially when working with complex JavaScript frameworks like Vue.js. As developers continue to adopt Vue.js for its flexibility and simplicity, understanding the tools available for effective debugging is crucial. In this article, we’ll delve into some of the best tools and techniques for debugging Vue.js applications.
Vue Devtools
One of the most powerful tools for Vue.js developers is the Vue Devtools. This browser extension is indispensable for inspecting and debugging Vue applications. With Vue Devtools, you can:
- Inspect Component Hierarchy: Understand your application structure with a tree view of all components.
- View Component Data: Examine data and props of each component in real-time.
- Time Travel Debugging: Go back and forth in your application’s state history.
- Performance Monitoring: Analyze and optimize your application’s performance.
Vue Devtools is available for Chrome and Firefox.
Console Logging
A fundamental technique that should not be underestimated is Console Logging. Using console.log()
can give immediate insight into the functioning of your code. While not as sophisticated as other tools, it’s sometimes the quickest way to identify the source of an issue.
Vue.js Error Handling
Vue.js provides built-in error handling and emitting events when errors occur, which can be used to capture and handle errors globally. This can be set up in your main.js
via the global error handler:
Vue.config.errorHandler = function (err, vm, info) {
// Handle the error
console.error(err);
};
Browser Developer Tools
Modern browsers come with powerful Developer Tools that can be utilized to debug JavaScript applications. Features like breakpoints, network inspection, and real-time DOM updates are beneficial when working with Vue.js.
ESLint and Vue ESLint Plugin
A linting utility like ESLint can prevent bugs by enforcing code standards and highlighting potential errors in your codebase. For Vue.js, the eslint-plugin-vue
provides specific linting rules geared towards Vue applications, ensuring you adhere to best practices.
Visual Studio Code Extensions
For those using Visual Studio Code as their IDE, a few extensions can significantly improve the development experience:
- Vetur: Offers syntax-highlighting, IntelliSense, and snippets for
.vue
files. - ESLint: Integrates ESLint directly into VS Code, highlighting issues as you code.
Additional Resources
If you’re keeping up with the latest enhancements in Vue.js, you might find this thread on recent Vue 3 updates useful. Additionally, for those exploring specific functionalities, these resources on conditional rendering in Vue.js and updating iframes in Vue.js could prove very helpful.
Conclusion
Whether you’re a seasoned veteran or just starting with Vue.js, having the right tools at your disposal is essential for efficient debugging. With tools like Vue Devtools, native error handling, and various IDE extensions, developers can streamline their debugging process and improve code quality. As the Vue.js ecosystem grows, staying informed about new tools and techniques remains crucial to successful development.
Make sure to explore and integrate these tools into your workflow to handle any bugs swiftly and continue building powerful applications with Vue.js.
Feel free to copy and paste this markdown content into your editor or platform that supports markdown rendering to see the formatting in action.