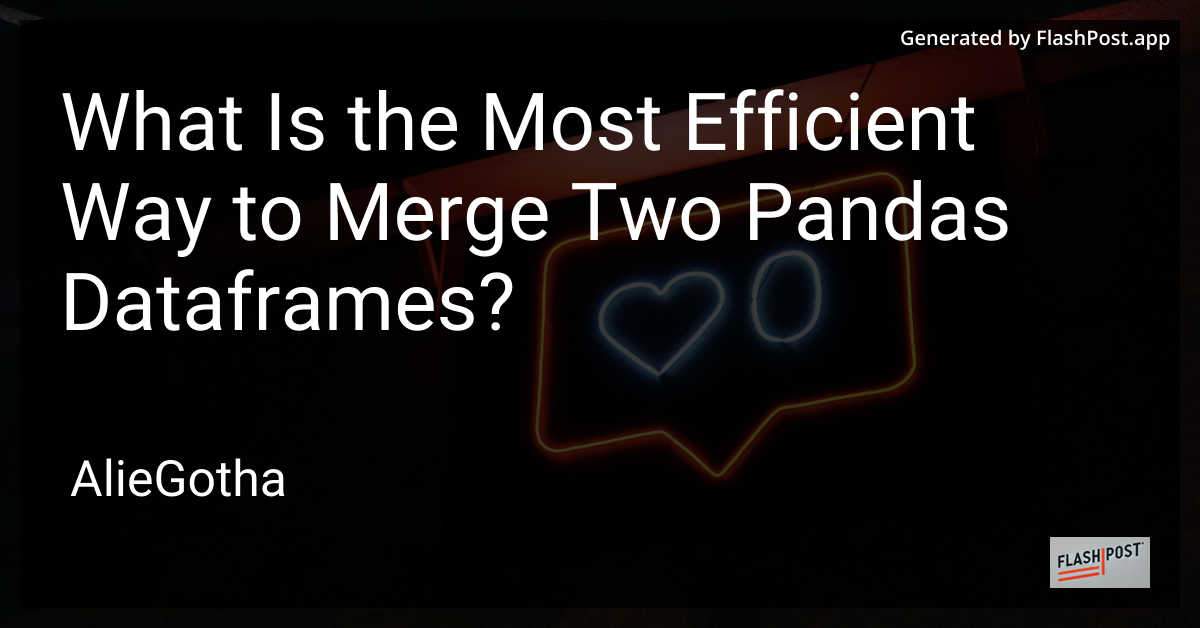
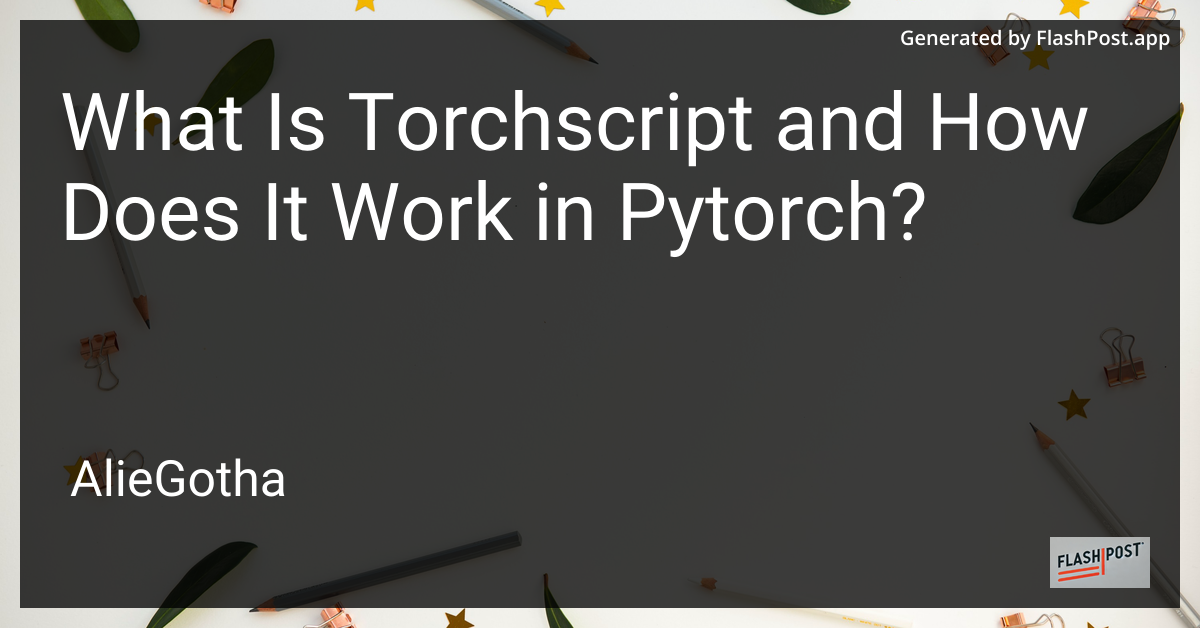
What Is Torchscript and How Does It Work in Pytorch?
PyTorch has become a leading deep learning framework, popular for its dynamic computation graph and ease of use. Among its many features, TorchScript offers a unique way to optimize and deploy PyTorch models across different platforms. In this article, we’ll dive into what TorchScript is, how it functions within the PyTorch ecosystem, and highlight its significance in enhancing model performance.
What is TorchScript?
TorchScript is an intermediate representation of a PyTorch model that can be run in a standalone C++ runtime environment. It allows for the conversion of a PyTorch model written in Python into a form that can be executed independently from Python. This capability is particularly useful for deploying models in production environments where Python may introduce dependencies or latency issues.
Key Features of TorchScript:
- Portability: TorchScript code can be exported from Python and then run in a C++ interpreter, making it suitable for deployment in environments that require models to be executed without Python.
- Efficiency: The serialized TorchScript code can often be executed more efficiently, optimizing both CPU and GPU performance.
- Interoperability: It promotes integration with other libraries or systems that utilize C++ as their primary language.
How Does TorchScript Work?
TorchScript operates through two main mechanisms in PyTorch:
-
Tracing: This involves running the model once with sample inputs and recording the operations that are executed. It’s a non-intrusive way to capture the model’s structure but may miss some dynamic computations that aren’t executed during tracing.
-
Scripting: This converts the entire model into TorchScript, capturing both operations and control flow. It requires defining the model using the TorchScript
@torch.jit.script
decorator, ensuring all model parts are convertible.
Steps to Convert a PyTorch Model to TorchScript
-
Tracing Example:
import torch import torch.nn as nn class MyModel(nn.Module): def forward(self, x): return x * 2 model = MyModel() example_input = torch.tensor([1.0]) traced_model = torch.jit.trace(model, example_input)
-
Scripting Example:
import torch @torch.jit.script def scripted_function(x): if x > 0.5: return x * 2 else: return x / 2 # Running the scripted function scripted_module = scripted_function(torch.tensor(0.4))
Practical Applications of TorchScript
TorchScript is invaluable for deploying models across diverse environments or platforms. Here are some practical applications:
- Model Combination: Combining multiple trained models into a cohesive framework. Explore more about model combination using PyTorch.
- Logging and Adjustment: For debugging and optimization purposes, printing intermediary results is essential. Learn about printing in PyTorch.
- Pre-trained Model Inference: Utilize TorchScript with pre-trained models to streamline the inference process. Check out how to make predictions with pretrained models in PyTorch.
- Customization: Create custom batched functions seamlessly with TorchScript for enhanced computation. Find out how to write a custom PyTorch function.
Conclusion
TorchScript is a powerful extension of PyTorch that transforms models for high-performance deployment. By making models portable and efficient, it enables seamless integration into production environments. Whether through tracing or scripting, TorchScript ensures that models are both agile and ready for execution on any platform, contributing significantly to the universality and scalability of PyTorch applications.
This SEO-optimized article is designed to inform readers on TorchScript while incorporating internal links related to advanced PyTorch topics.