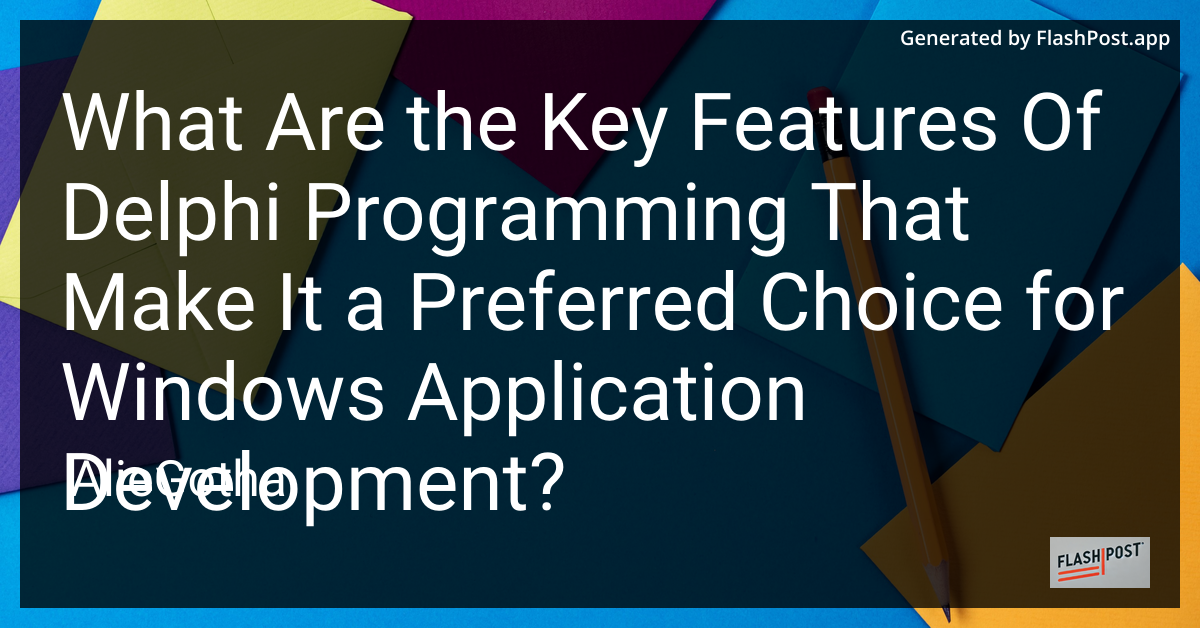
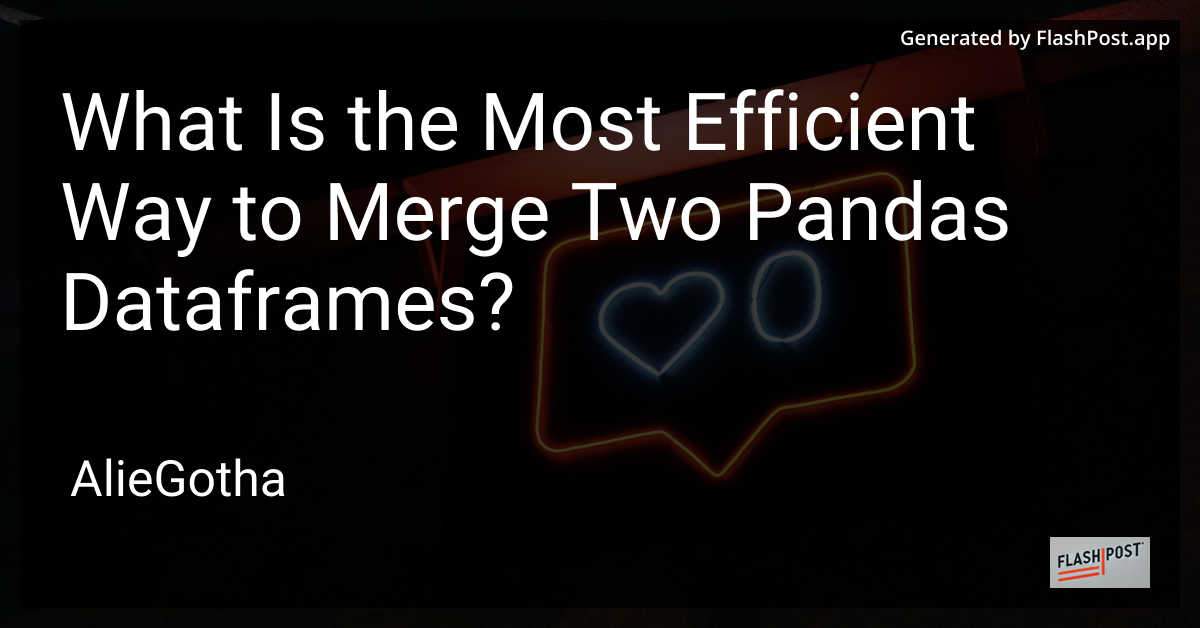
What Is the Most Efficient Way to Merge Two Pandas Dataframes?
Merging dataframes is a common task for data scientists and analysts working with Pandas DataFrames. It allows you to combine information from different sources into one comprehensive dataset, facilitating analysis and insights. But with numerous methods available to perform the merge, what is the most efficient way? Let’s explore the most optimal approach to merging two Pandas DataFrames.
Understanding Merging in Pandas
Before diving into the efficient methods, it is crucial to understand what merging entails. Merging, in the context of Pandas, is the combination of two datasets along their shared columns or indices using a logic similar to SQL joins. This process forms a new DataFrame which relies on the intersection of data sharing a common identifier or key.
Efficient Merging Techniques
1. Utilize merge()
Function
The most efficient and versatile option for merging two DataFrames is the merge()
function. The syntax is intuitive, and it performs efficiently with large datasets, given its C implementation in Pandas.
import pandas as pd
df1 = pd.DataFrame({'key': ['A', 'B', 'C'], 'value1': [1, 2, 3]})
df2 = pd.DataFrame({'key': ['A', 'B', 'D'], 'value2': [4, 5, 6]})
result = pd.merge(df1, df2, on='key', how='inner')
In the example above, df1
and df2
are merged on the key
column using an inner join, which is the default how
parameter.
2. Choose the Right Merge Type
Selecting the appropriate merge type is critical for efficiency and ensuring data integrity:
inner
: Returns rows with keys present in both DataFrames.outer
: Includes all keys from both, filling with NaNs where one DataFrame lacks a matching key.left
: Encompasses all keys from the left DataFrame, with corresponding entries from the right.right
: Comprises all keys from the right DataFrame, with respective entries from the left.
3. concat()
vs. merge()
While both functions are used to combine DataFrames, using merge()
is recommended when dealing with datasets sharing unique keys or indices. The performance benefit becomes more evident in large-scale datasets due to its optimized C implementation.
Learn more about comparing DataFrame merge operations.
4. Efficient Filtering Before the Merge
To optimize performance, always consider filtering data before a merge operation. By narrowing down DataFrames to relevant parts, you reduce memory usage and speed up the operation. Check out how to filter on string columns using a between clause.
Conclusion
Merging DataFrames efficiently requires an understanding of the tools available in Pandas and employing strategies that enhance performance. With the merge()
function, you can perform different join operations efficiently. Always ensure you use the appropriate join type and preprocess DataFrames through filtering to optimize the performance further.
To expand your expertise in manipulating DataFrames with Pandas, visit the following resources:
With these insights, you can perform optimized and efficient data merging operations, unlocking the full potential of your datasets in Python.