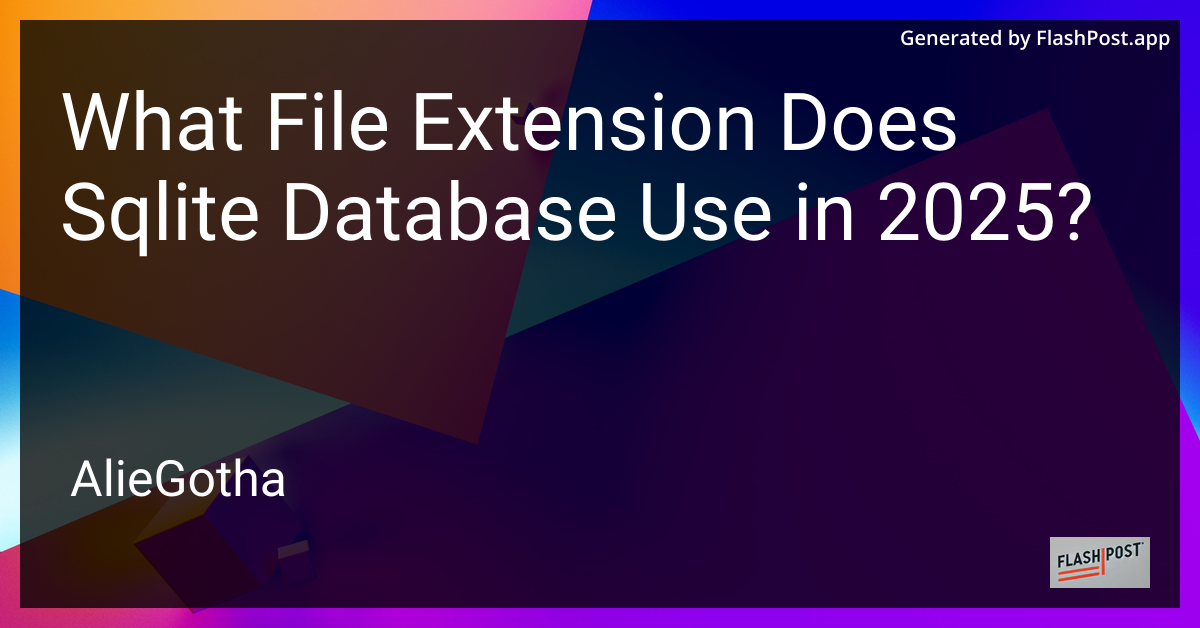
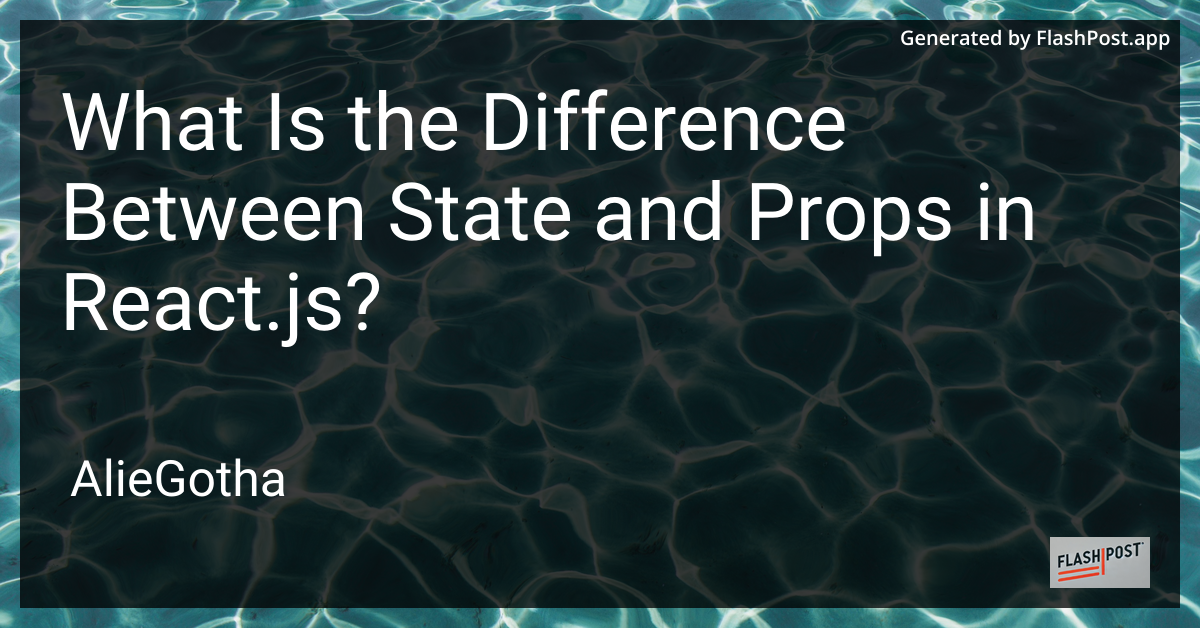
What Is the Difference Between State and Props in React.js?
React.js, a popular JavaScript library for building user interfaces, introduces two critical concepts that developers frequently work with: state and props. While state and props might initially seem similar, they serve different purposes in React components. In this article, we explore these differences and help you understand when to use each.
What is State in React.js?
State is an object in a React component that holds dynamic data that influences the component’s behavior and rendering. Unlike props, state is managed within a component and can change over time, usually in response to user actions or system events. Here are some key characteristics of state:
- Local and Encapsulated: State is encapsulated in the component where it is defined. It can only be accessed and modified within that component.
- Mutable: State can be updated over time. When you update the state, React re-renders the component to reflect the new data.
- Controlled by the Component: A component is responsible for updating its state using the
setState
function in class components or theuseState
hook in functional components.
What are Props in React.js?
Props, short for properties, are used to pass data from one component to another. They are read-only and immutable, meaning a component cannot modify the props it receives. Below are some important aspects of props:
- Read-Only: Props are immutable. Once set by the parent, a component cannot alter its own props.
- Passed Down the Component Tree: Data is passed from parent to child components through props, enabling communication between components.
- Stateless Data: Since props do not change within a component, they represent a fixed snapshot of data tied to a specific render cycle.
Key Differences Between State and Props
-
Mutability: State is mutable, meaning it can change over time. Conversely, props are immutable.
-
Ownership:
- State: Owned and managed by the component itself.
- Props: Owned by the parent component (the component that renders another component and passes down props).
-
Purpose:
- State: Used for data that a component will mutate, typically as a response to user events.
- Props: Used for passing data and callback functions down the component tree.
Practical Implications
Deciding when to use state or props depends on the component’s role in your application:
- Use State: When the component needs to maintain or track changes internally. Typical examples include form input fields, toggles, and any data that changes in response to user actions.
- Use Props: When you want to pass data to child components or when you need to trigger functions in parent components (such as callback functions).
Further Reading and Resources
- Learn about setting up your React.js application on A2 Hosting by following this handy guide.
- Explore how to launch a React.js application using Rackspace for more deployment options.
- Discover techniques to optimize iframes in React.js for improved performance.
- Integrate GraphQL with React.js to enhance your application’s data handling capabilities.
Understanding the distinction between state and props is fundamental to mastering React.js and crafting effective user interfaces. By leveraging these tools appropriately, you can create dynamic, reactive, and maintainable applications.