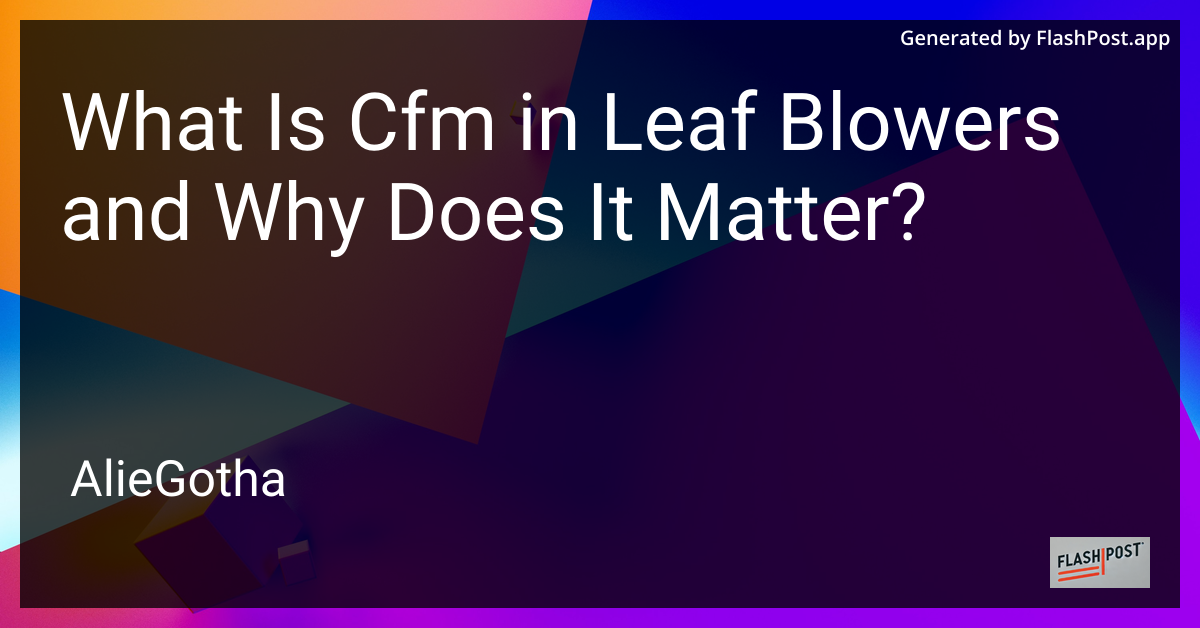
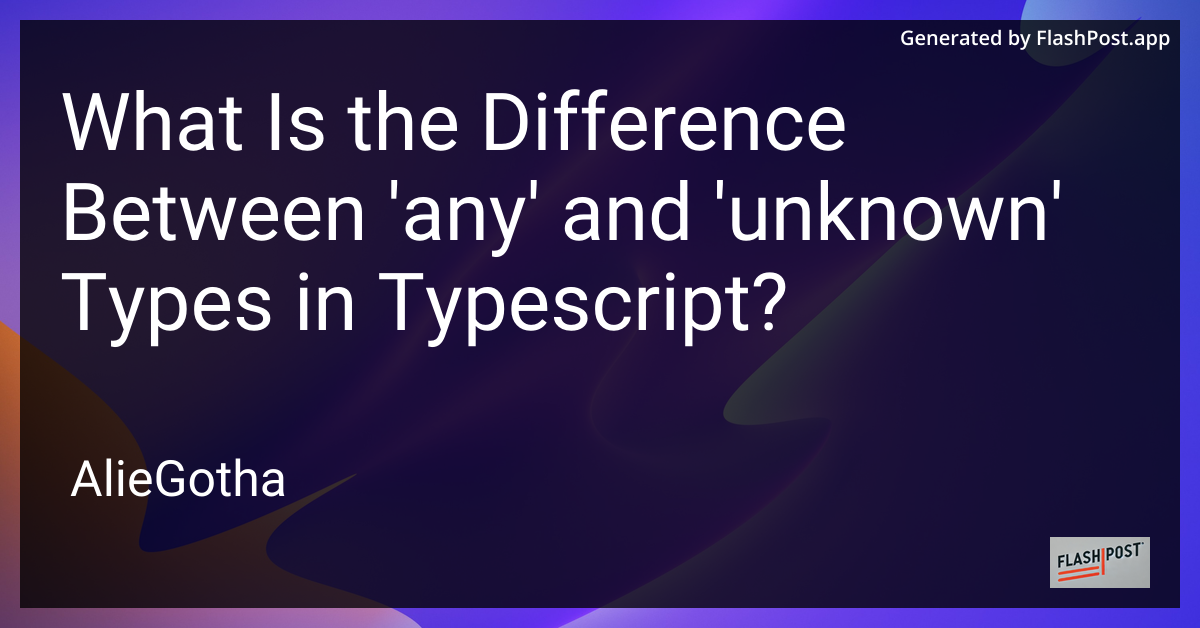
What Is the Difference Between 'any' and 'unknown' Types in Typescript?
TypeScript, a popular superset of JavaScript, adds robust typing capabilities to JavaScript, making it easier to write and manage large codebases. Among its many features, TypeScript introduces special types like any
and unknown
which are essential for dynamic type checking. In this article, we will explore the key differences between any
and unknown
types, and why knowing these differences is crucial for maintaining type safety in your TypeScript projects.
What is the any
Type?
In TypeScript, the any
type is a wildcard type that allows any and all operations to be performed on it without any type-checking. Essentially, using any
bypasses TypeScript’s compile-time type checking, granting you maximal flexibility. This comes in handy when migrating JavaScript codebases to TypeScript or when interfacing with third-party libraries with unknown types. However, excessive use of any
reduces type safety, potentially leading to runtime errors.
Example of Using any
:
let randomValue: any = 10;
randomValue = "Hello";
randomValue = true;
// The above assignments cause no errors with 'any'
let sum = randomValue + 10; // Valid, but might cause a runtime error
What is the unknown
Type?
The unknown
type is TypeScript’s type-safe counterpart to any
. It signifies that a variable could be of any type, but unlike any
, you cannot perform operations on an unknown
type without first performing a type check or an assertion. This restriction ensures that developers consciously validate the type before using it, thereby enforcing type safety.
Example of Using unknown
:
let uncertainValue: unknown = 10;
uncertainValue = "World";
uncertainValue = false;
// Type checks or assertions are required before operations
if (typeof uncertainValue === "string") {
console.log(uncertainValue.toUpperCase()); // Safe to perform string operations
}
Key Differences Between any
and unknown
-
Type Safety:
any
: Offers no compile-time type safety and permits any operation.unknown
: Provides type safety by enforcing type checks before usage.
-
Flexibility:
any
: Extremely flexible and permissive.unknown
: Requires checks and is more restrictive.
-
Inferences and Assignments:
any
: Can be easily assigned to other types without restriction.unknown
: Requires explicit type assertions or checks for assignment to other types.
-
Use Cases:
any
: Useful in scenarios where type-checking is temporarily undesirable or infeasible.unknown
: Ideal when you receive data from untrusted sources, ensuring you validate the type before use.
Conclusion
Understanding the difference between any
and unknown
is essential for writing reliable TypeScript code. While any
offers flexibility at the cost of potential runtime errors, unknown
provides a safer alternative by enforcing type checks. Use unknown
wherever possible to keep your TypeScript code robust and error-free.
For further exploration of TypeScript concepts, take a look at these resources:
- Compute momentum using TypeScript formulas
- Techniques for TypeScript type casting
- Handling promises and asynchronous programming in TypeScript
By embracing these concepts and best practices, you can enhance both the maintainability and safety of your TypeScript projects.