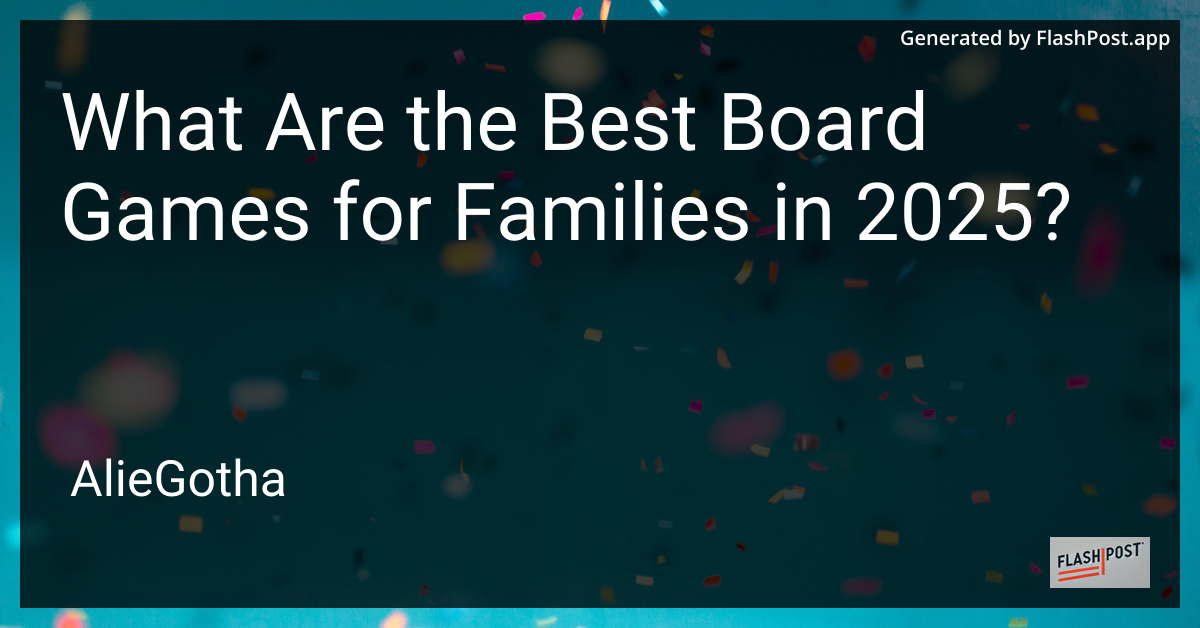
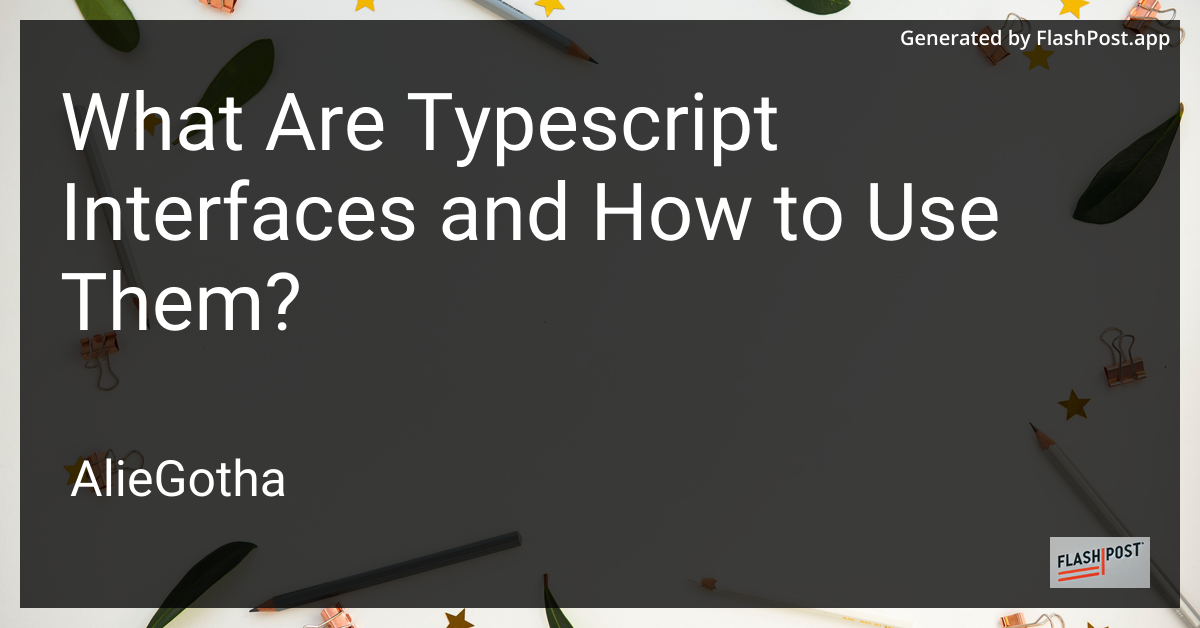
What Are Typescript Interfaces and How to Use Them?
TypeScript, a powerful extension of JavaScript, has greatly improved the way we write and manage code. One of the most crucial features in TypeScript is interfaces. They are essential for robust and scalable applications. In this article, we’ll explore what TypeScript interfaces are and how to effectively use them in your projects.
What are TypeScript Interfaces?
In TypeScript, an interface is a syntactical contract that defines the shape of an object. Interfaces define properties and methods that an object should implement without specifying the concrete implementation. They are used to define custom types, making your code reusable and easier to maintain.
Key Features of Interfaces
- Type Checking: Interfaces provide a way to enforce compile-time checks, ensuring that objects adhere to a specific structure.
- Code Readability: By documenting the expected structure of objects, interfaces enhance code readability and comprehension.
- Reusability: Interfaces can be reused across multiple components or modules, promoting DRY (Don’t Repeat Yourself) principles.
How to Use Interfaces in TypeScript
Defining an Interface
An interface in TypeScript can be defined using the interface
keyword followed by the structure. Here’s an example:
interface User {
id: number;
name: string;
email: string;
isActive: boolean;
}
Implementing an Interface
An interface can be implemented by objects to ensure they conform to its specifications. Here’s how an interface can be utilized:
const user: User = {
id: 1,
name: "John Doe",
email: "john.doe@example.com",
isActive: true,
};
Function Interfaces
Interfaces can also define the shape of functions:
interface Greeting {
(name: string): string;
}
const sayHello: Greeting = (name: string) => `Hello, ${name}!`;
Extending Interfaces
Interfaces can extend other interfaces, allowing them to inherit properties and methods:
interface Admin extends User {
permissions: string[];
}
const admin: Admin = {
id: 1,
name: "Jane Smith",
email: "jane.smith@example.com",
isActive: true,
permissions: ["read", "write"],
};
Advanced Usage of Interfaces
- Optional Properties: Use the
?
symbol to denote optional properties. - Read-Only Properties: Use the
readonly
modifier to prevent modification after initialization.
Conclusion
Interfaces are a foundational element in TypeScript, aiding in creating clear and well-structured applications. Through type checking, they ensure that your code is robust and less prone to runtime errors.
For more information on other TypeScript functionalities, you might be interested in:
- Conditionally Skipping Mocha Tests in TypeScript
- Converting or Running TypeScript as JavaScript
- Printing iFrame Contents in TypeScript
- Building an Online Community with TypeScript and NestJS
By leveraging TypeScript interfaces, developers can build more reliable, maintainable, and scalable applications with greater ease.