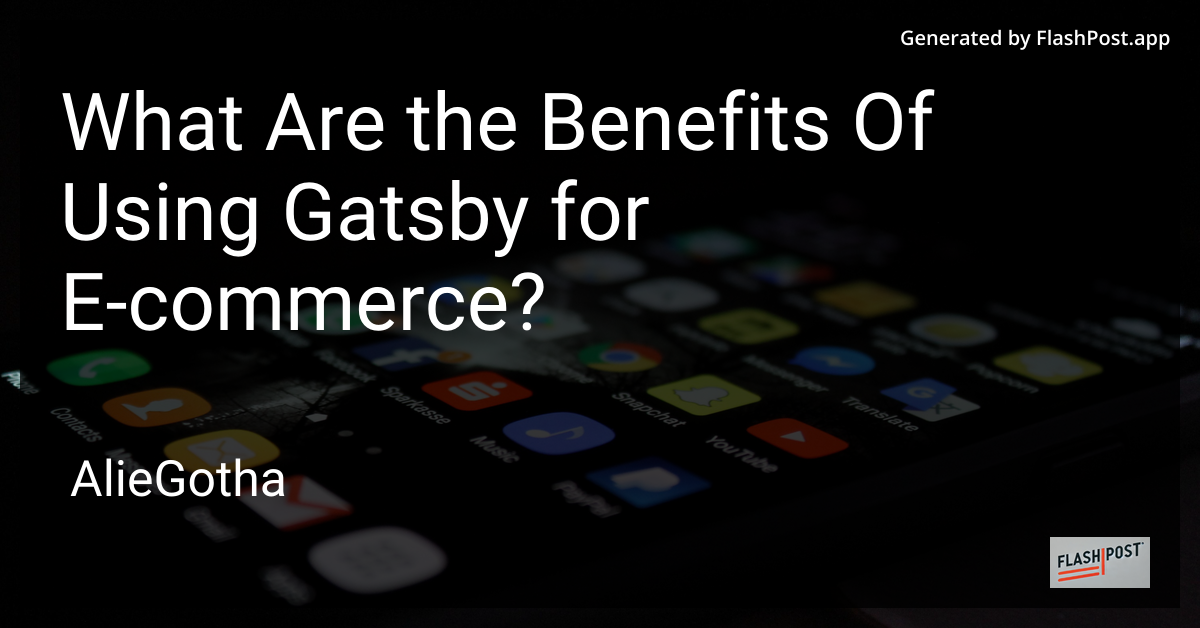
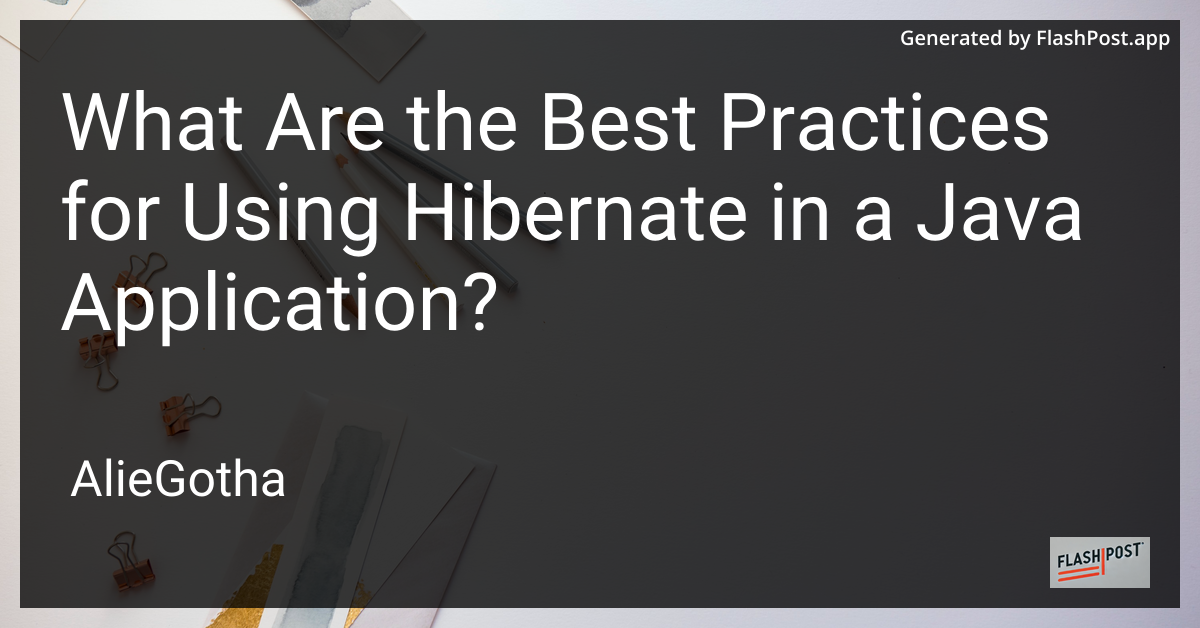
What Are the Best Practices for Using Hibernate in a Java Application?
Hibernate is a powerful Object-Relational Mapping (ORM) tool for Java applications, streamlining database operations by abstracting complexities. However, leveraging Hibernate effectively requires adherence to best practices to ensure performance, maintainability, and scalability. This article explores some of the top best practices for using Hibernate in your Java applications.
1. Use Lazy Loading Wisely
Lazy loading is a Hibernate feature that delays the initialization of an object until it’s necessary. While it can improve performance by reducing the initial load time and memory consumption, improper use might lead to LazyInitializationException
. To leverage lazy loading effectively, ensure session objects are open when accessing related entities, or use FetchType.EAGER
for critical relationships. For more on managing relationships, check out this guide on mapping a foreign key with Hibernate.
2. Optimize Your HQL/JPQL Queries
Hibernate Query Language (HQL) and Java Persistence Query Language (JPQL) offer flexibility in querying data. However, inefficient queries can significantly affect application performance. Always:
- Avoid SELECT N+1 Problem: Use joins or fetch profiles to retrieve related entities.
- Use Pagination: For large datasets, utilize
setFirstResult()
andsetMaxResults()
to limit results. - Index Analysis: Ensure database indexes align with the queries being executed.
3. Caching Strategies
Caching can dramatically improve application performance by reducing database access. Hibernate provides first-level and second-level caching solutions:
- First-level cache: Default for a session, ensuring queries within a session are minimized.
- Second-level cache: Enables shared cache across sessions. Consider implementing EHCache or Infinispan for frequent read operations.
4. Transaction Management
Proper transaction management is critical. Always encapsulate database operations within transactions, either programmatically or using declarative approaches like annotations. Consider using frameworks like Spring for comprehensive transaction management capabilities, ensuring consistent and atomic transactions across services.
5. Use Connection Pools
Efficient connection handling is essential in Hibernate applications. Configure a connection pool provider like C3P0 or HikariCP to manage database connections effectively, improving response times and resource management. For configuring connections, refer to this detailed article on getting Oracle connections from Hibernate.
6. Monitor and Tune Performance
Regularly monitor your Hibernate application’s performance. Use tools like Hibernate’s statistics feature to track query execution times, cache hit rates, and other metrics. Profiling and logging can also identify bottlenecks, paving the way for targeted optimizations.
7. Schema Management
Managing database schema changes is critical, especially in production environments. Hibernate’s auto-update feature (hbm2ddl.auto
) is useful for development but avoid using it in production. Instead, consider generating migrations using tools like Flyway or Liquibase. Learn how to generate migrations with Hibernate for robust schema management.
Conclusion
Employing these best practices for using Hibernate in a Java application aids in building robust, high-performance, and scalable applications. By carefully managing how your application interacts with the database and leveraging Hibernate’s powerful features, you can ensure efficient database operations and a smoother development process.
For further insights into managing specific Hibernate functionality, explore topics on foreign key relationships, connection handling, and database migrations.
This article provides foundational insights and encourages continued exploration to tailor strategies to specific application needs.