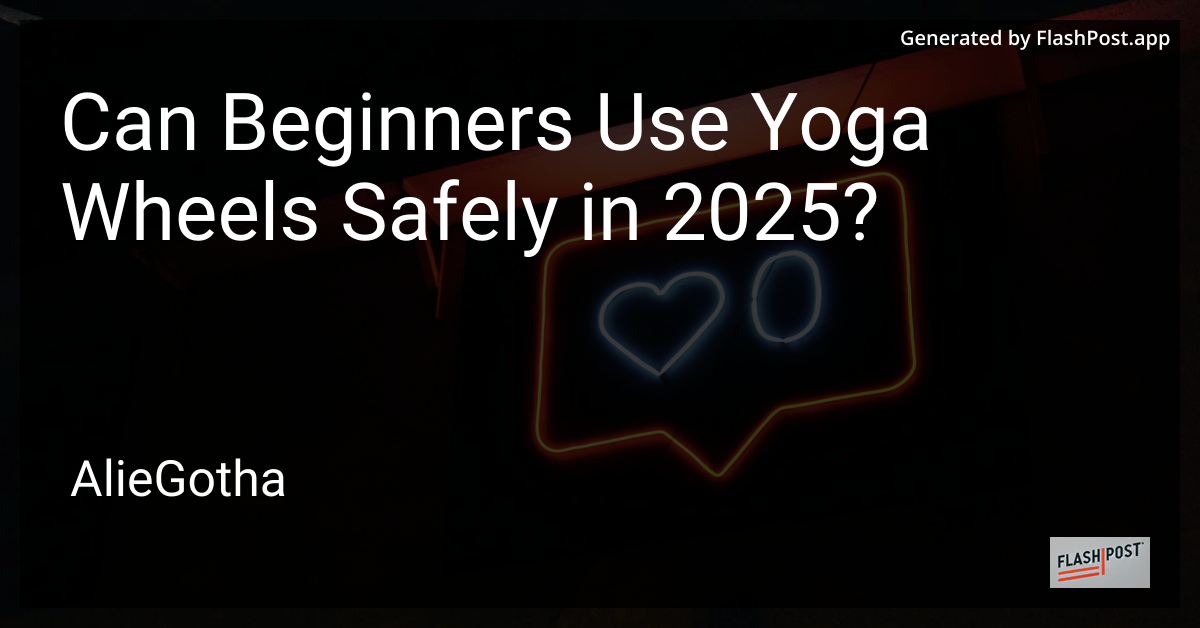
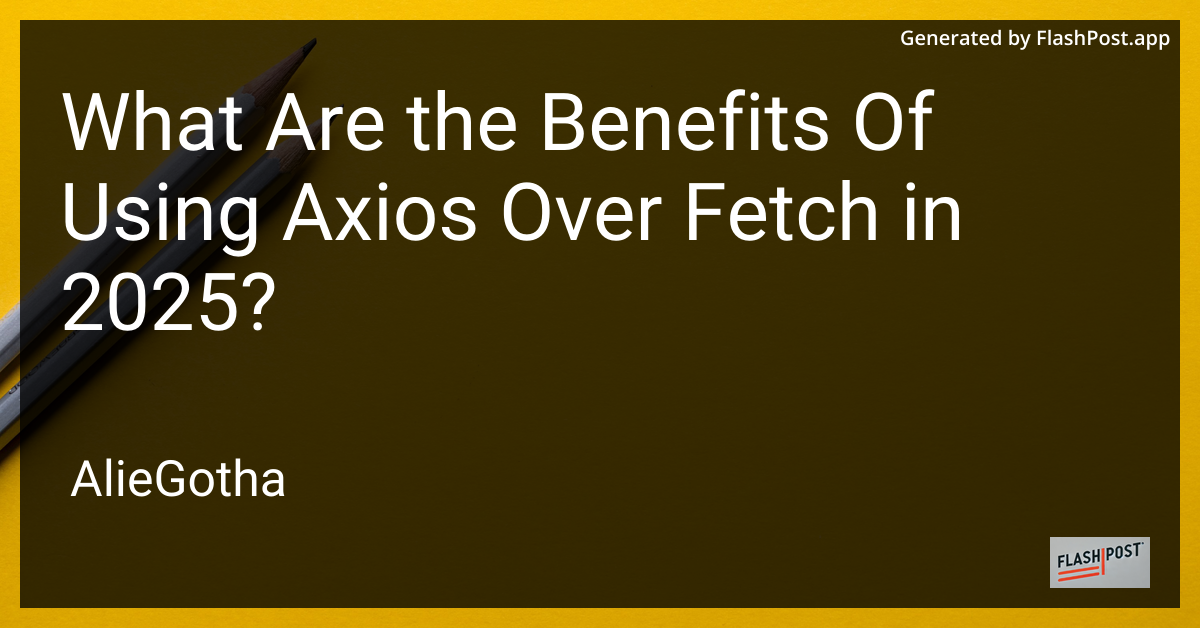
What Are the Benefits Of Using Axios Over Fetch in 2025?
In the ever-evolving landscape of JavaScript development, making informed choices about the tools and libraries you use is crucial for maximizing efficiency and performance. While the Fetch API has been the standard for making HTTP requests in modern browsers, Axios continues to hold its ground with robust features that can greatly benefit developers in 2025. Below, we explore the advantages of using Axios over Fetch and how they can enhance your development workflow.
Why Choose Axios?
1. Simplified Syntax
Axios offers a more concise and intuitive syntax compared to Fetch, which can make your code cleaner and easier to read. For instance, handling JSON data in Fetch requires additional steps to parse, whereas Axios automatically transforms JSON, saving you from extra boilerplate code.
// Fetch example
fetch(url)
.then(response => response.json())
.then(data => console.log(data));
// Axios example
axios.get(url)
.then(response => console.log(response.data));
2. Interceptors for Requests and Responses
One of Axios’s powerful features is its ability to intercept requests or responses before they are handled by then()
or catch()
. This enables you to apply global transformations or handle errors in a centralized manner, which is invaluable for maintaining a clean codebase.
axios.interceptors.request.use(request => {
console.log('Starting Request', request);
return request;
});
axios.interceptors.response.use(response => {
console.log('Response:', response);
return response;
});
3. Automatic JSON Data Transformation
With Axios, received JSON data is automatically transformed, whereas with Fetch, you need to manually convert the stream into a JSON object. This automatic processing in Axios saves time and reduces the likelihood of errors.
4. Better Error Handling
Axios provides improved error handling. When you make a request using Fetch, you always receive a resolved promise unless there’s a network error. In contrast, Axios treats even HTTP errors as errors, returning a rejected promise. This makes it easier to handle failed responses directly in your catch()
block.
5. Cancelation of Requests
Axios supports request cancellation, which is extremely useful in scenarios like component unmounting in React or when requests are no longer needed. Although Fetch introduced the AbortController API, Axios wraps this functionality in a simpler interface.
const CancelToken = axios.CancelToken;
const source = CancelToken.source();
axios.get(url, {
cancelToken: source.token
}).catch(thrown => {
if (axios.isCancel(thrown)) {
console.log('Request canceled', thrown.message);
} else {
// handle error
}
});
// Cancel the request
source.cancel('Operation canceled by the user.');
6. Wide Browser Support
While browser support for Fetch has improved significantly, Axios still ensures compatibility across a broader range of environments, making it a safer choice for supporting a diverse set of users.
Conclusion
In 2025, Axios retains its place as a valuable tool for developers looking for an efficient, feature-rich HTTP client. Its user-friendly syntax, superior error handling, built-in request/response interception, and additional features set it apart from Fetch. By adopting Axios, you can streamline your development process and maintain a cleaner codebase.
For more on using JavaScript effectively in modern web development, check out these related articles:
- JavaScript Framework: Learn how to add a click event on Vuetify headers.
- JavaScript Redirect: Explore how to use multiple redirects with one JavaScript function.
- JavaScript iFrame Function Call: Discover techniques for calling a function from an iframe.
By staying informed about the latest in JavaScript tools and techniques, you can ensure your projects are both modern and efficient.
This article is intentionally structured with SEO best practices, including a clear heading structure, keyword usage, and internal and external links for enhanced visibility in search engines.