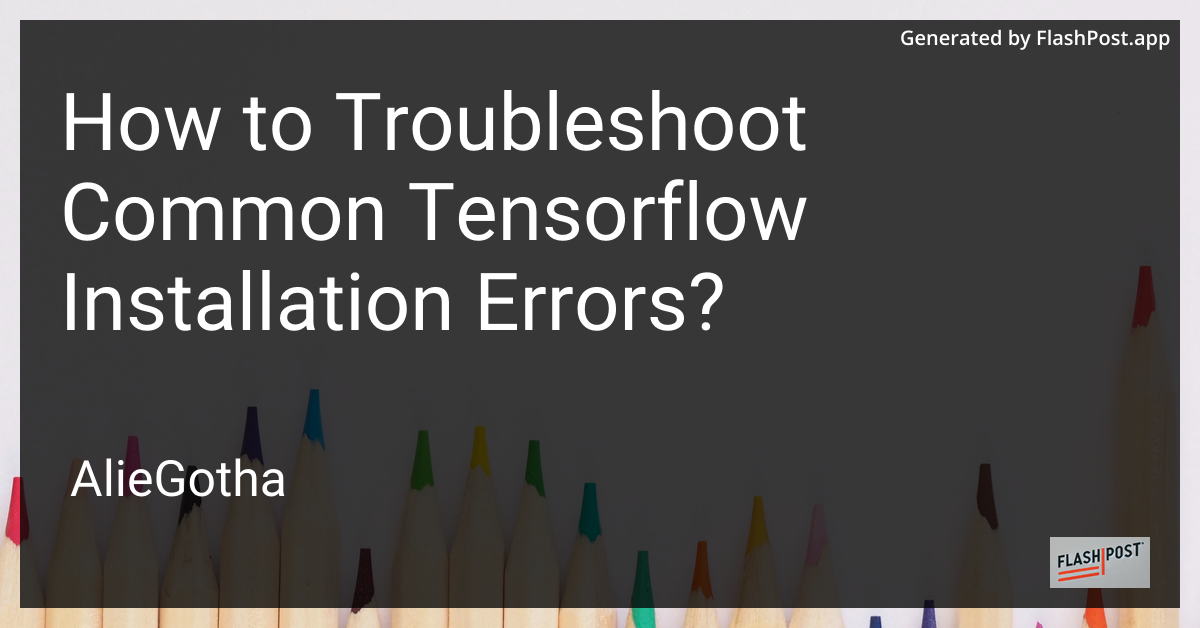
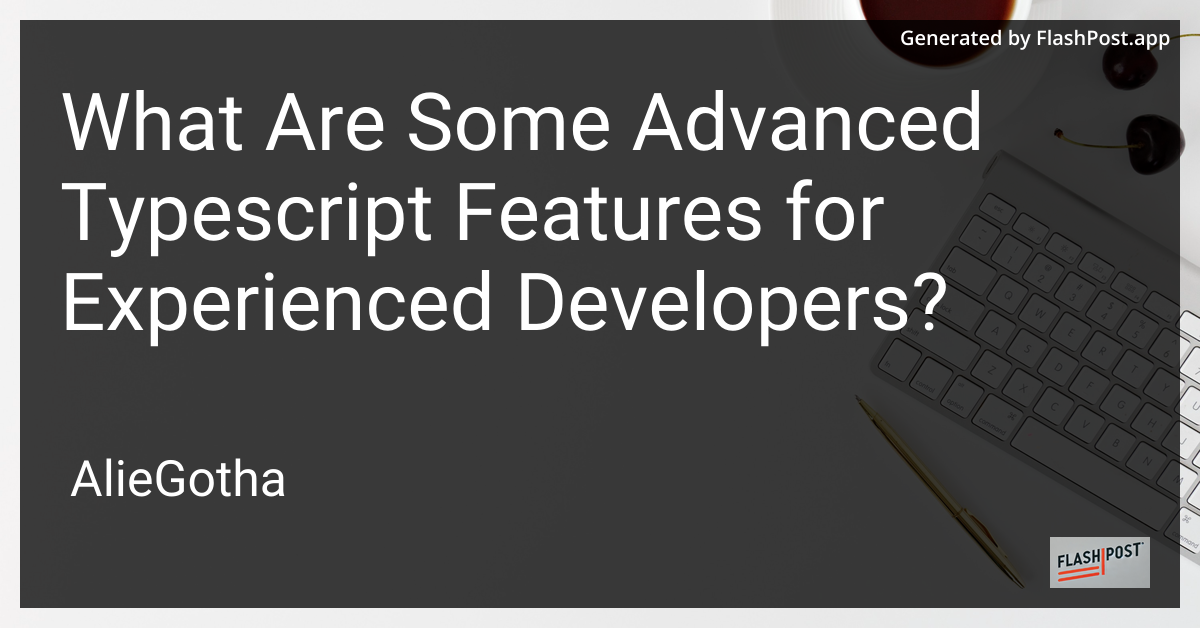
What Are Some Advanced Typescript Features for Experienced Developers?
TypeScript has become an essential tool for developers who want to reduce bugs, improve code quality, and leverage modern JavaScript features with type-checking capabilities. While many developers are familiar with basic TypeScript features, its true potential lies in advanced functionalities that can greatly enhance productivity and code robustness. In this article, we’ll explore some advanced TypeScript features that experienced developers should master.
Advanced Types
Intersection Types
Intersection types allow you to combine multiple types into one. This is particularly useful when you want an object to conform to multiple contracts.
interface Account {
name: string;
email: string;
}
interface Admin {
role: string;
}
type AdminAccount = Account & Admin;
Union Types with Exhaustive Checks
Union types can be paired with exhaustive checks to ensure that all possible values are handled, which can prevent runtime errors.
type Shape = "circle" | "square";
function getArea(shape: Shape): number {
switch (shape) {
case "circle":
return Math.PI * 1 * 1; // Assuming radius = 1
case "square":
return 1 * 1; // Assuming side = 1
default:
const _exhaustiveCheck: never = shape;
return _exhaustiveCheck;
}
}
Advanced Function Types
Overloaded Function Types
Overloading lets you define multiple versions of a function signature. This is valuable when a function can behave differently based on the input types.
function combine(input1: string, input2: string): string;
function combine(input1: number, input2: number): number;
function combine(input1: any, input2: any) {
if (typeof input1 === "string" && typeof input2 === "string") {
return input1 + input2;
} else if (typeof input1 === "number" && typeof input2 === "number") {
return input1 + input2;
}
}
Conditional Types
Conditional types allow types to be determined based on a condition.
type IsString<T> = T extends string ? "This is a string" : "Not a string";
let checkString: IsString<number>; // "Not a string"
Mapped Types
Mapped types let you transform properties in an existing type and create new types. They are especially useful for creating versatile utility types.
type Nullable<T> = {
[K in keyof T]: T[K] | null;
};
interface User {
id: number;
name: string;
}
type NullableUser = Nullable<User>;
Utility Types
TypeScript comes with several built-in utility types such as Partial
, Readonly
, and Record
. Understanding how to use and create custom utility types can significantly increase your efficiency.
interface Project {
title: string;
status: string;
}
const inProgress: Partial<Project> = {
status: "in-progress"
};
Mastering TypeScript for Enhanced Development
These advanced TypeScript features can significantly enhance your development experience by adding more type safety, reducing errors, and enhancing code readability. By mastering these features, you can write more maintainable and robust TypeScript code.
For further reading, you can explore more about running TypeScript as JavaScript, learn how to print contents within TypeScript, understand Fibonacci extensions in TypeScript, or delve into mocking Axios with Mocha.
By integrating these advanced features into your workflow, you enhance your expertise and leverage the full power of TypeScript for building modern applications.