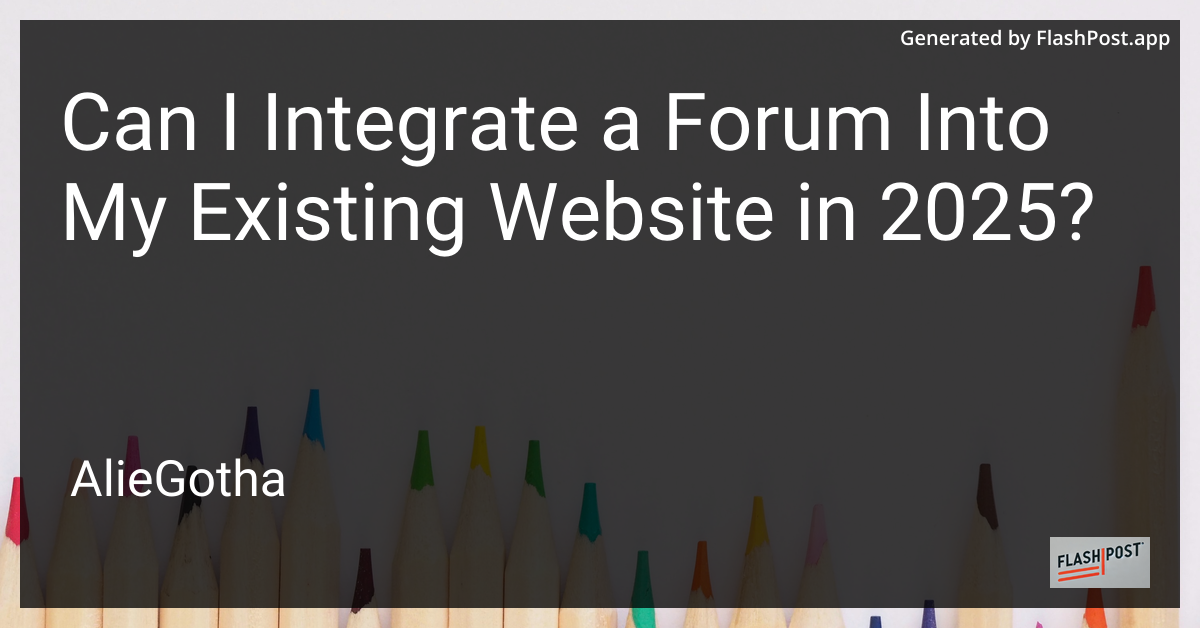
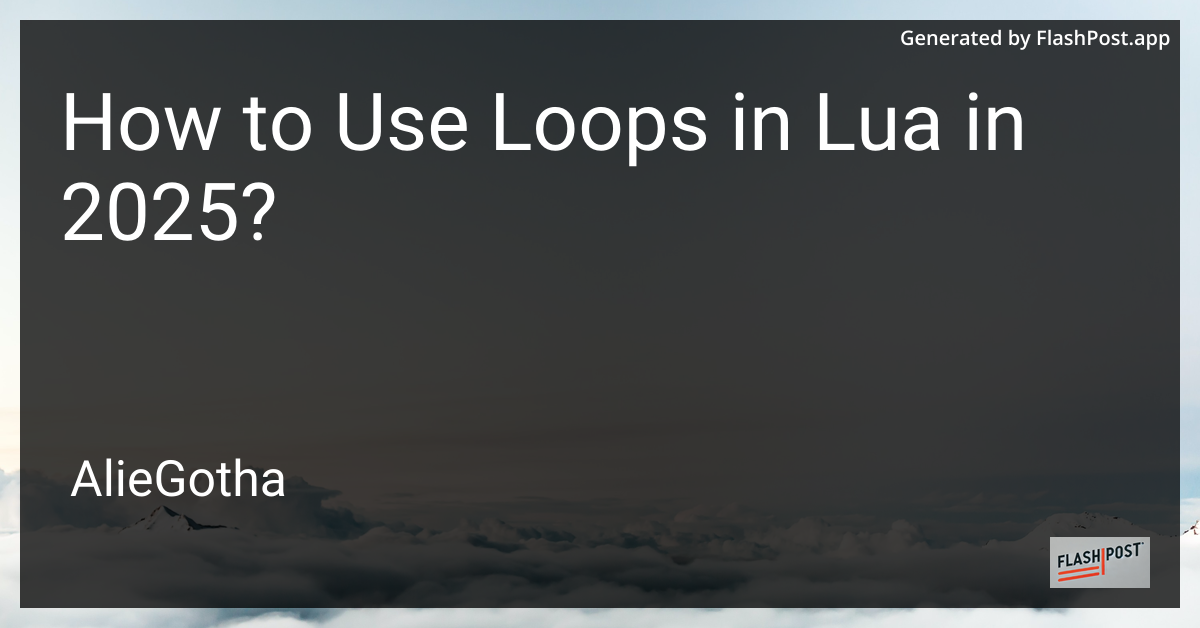
How to Use Loops in Lua in 2025?
title: Mastering Loops in Lua: A Guide to Efficient Coding in 2025 description: Learn how to effectively use loops in Lua with our comprehensive guide. Explore practical examples and tips to optimize your Lua coding skills in 2025. keywords: Lua, loops, programming, 2025, coding, software development, Lua guide
Loops are a fundamental concept in programming, allowing you to execute a block of code multiple times efficiently. In Lua, loops are versatile and powerful, making them an essential tool for any developer working with this lightweight programming language. In this article, we’ll explore how to use loops in Lua effectively, providing examples to enhance your coding in 2025.
Understanding Loops in Lua
Lua offers several loop constructs, each suited for different scenarios. The primary loop types in Lua are:
for
Loop: Ideal for numerical iterations, where you know the number of iterations in advance.while
Loop: Suitable when the number of iterations is not predetermined.repeat...until
Loop: Similar todo...while
loops in other languages, they always execute at least once.
Example of a for
Loop
The for
loop is straightforward and extremely useful when working with a defined range.
for i = 1, 10 do
print("Iteration: ", i)
end
In this example, the loop will run ten times, printing the iteration number during each pass.
Using the while
Loop
The while
loop evaluates its condition before each iteration, making it ideal for scenarios where the number of iterations depends on dynamic conditions.
local count = 0
while count < 10 do
print("Count is: ", count)
count = count + 1
end
This loop continues as long as count
is less than 10, incrementing count
each time.
Exploring repeat...until
Loop
The repeat...until
loop is useful when the block needs to be executed at least once, such as when waiting for user input.
local input
repeat
print("Enter a number (0 to quit):")
input = tonumber(io.read())
until input == 0
Here, the loop will ask the user to input a number repeatedly until they enter 0
.
Practical Loop Applications in Lua
Loops in Lua are often used to manipulate tables, a core data structure in Lua. You can visit our related guide on retrieving table length in Lua to gain more insights into table manipulation.
Additionally, integrating Lua with other systems often requires modules. For those working on a Linux environment, our guide on installing Lua modules on Linux can assist you with the setup.
Conclusion
Mastering loops in Lua is crucial for efficient coding. In 2025, as Lua continues to be utilized in various applications, understanding how to leverage loops effectively will enhance your software development capabilities. Whether it’s iterating over arrays or implementing conditional logic, loops are indispensable in optimizing your code.
By exploring practical examples and utilizing external resources, you can significantly improve your Lua programming skills. Happy coding!