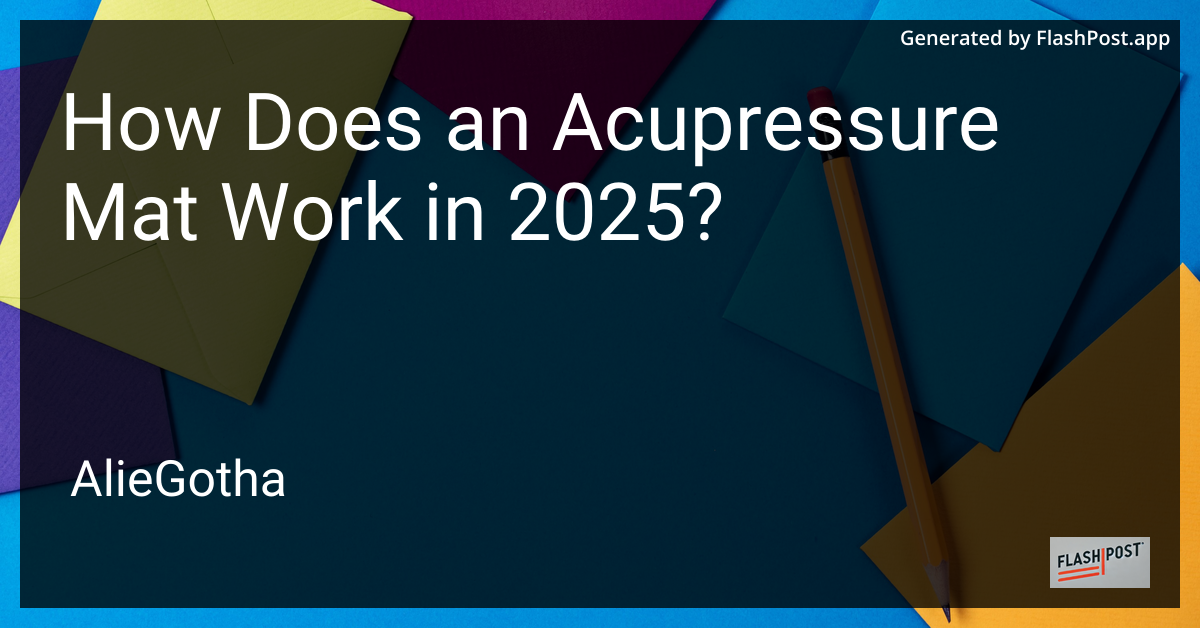
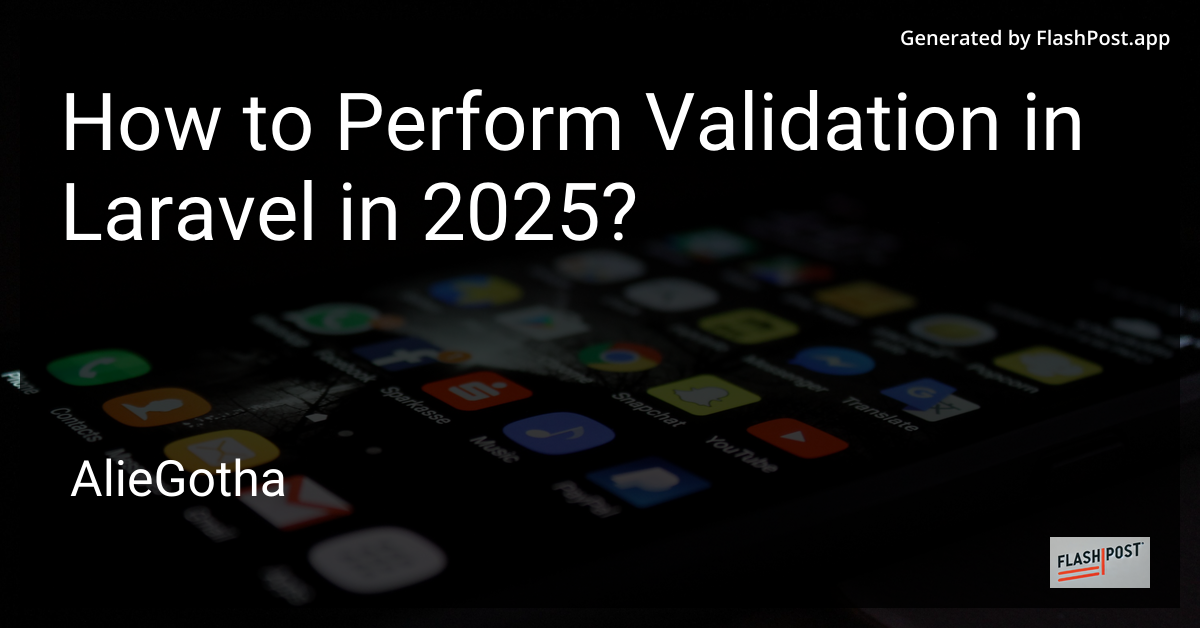
How to Perform Validation in Laravel in 2025?
In the ever-evolving landscape of web development, robust validation is essential for delivering secure and reliable applications. Laravel, a PHP framework, continues to be a preferred choice for developers due to its elegant syntax and powerful features. As we look forward to 2025, here’s a comprehensive guide on how to perform validation in Laravel while incorporating the latest best practices.
Why Validation Matters
Validation is crucial for ensuring that the data submitted by users meets your application’s expectations. It protects your application from invalid or potentially harmful inputs, and it’s a vital component of any secure web application.
Setting Up Laravel Validation
Laravel makes validation simple and intuitive. Let’s explore how to leverage Laravel’s validation features in 2025:
Step 1: Define Validation Logic
In Laravel, validation logic can be defined using several methods:
- Controller Method: Use the
validate
method directly in your controller actions. - Form Requests: Encapsulate validation logic in custom request classes.
- Manually Using Validator: Create an instance of the
Validator
and apply custom rules.
Step 2: Validation Rules
Laravel offers a wide array of validation rules. Some popular rules include required
, unique
, email
, min
, max
, and regex
. Here’s an example:
$request->validate([
'username' => 'required|string|max:255',
'email' => 'required|email|unique:users,email',
'password' => 'required|min:8|confirmed',
]);
For more complex scenarios, you can use custom validation rules or even use closures within your rules.
Step 3: Error Messages
By default, Laravel provides error messages. However, you can customize these messages if necessary:
$request->validate([
'username' => 'required',
], [
'username.required' => 'Please enter your username.',
]);
Step 4: Conditional Validation
Laravel’s validation can dynamically change rules based on the request data or any condition:
$request->validate([
'password' => function($attribute, $value, $fail) use ($request) {
if ($value !== $request->input('password_confirmation')) {
$fail('The password confirmation does not match.');
}
},
]);
Step 5: Validating Arrays and Files
Laravel handles complex data types efficiently, such as arrays and files:
$request->validate([
'photos.*' => 'image|mimes:jpeg,png,jpg,gif,svg|max:2048',
]);
Step 6: Handling Validation Failures
When validation fails, Laravel automatically redirects back to the previous page with errors stored in the session. Customize handling by returning JSON responses or redirecting to specific routes.
Useful Resources
Conclusion
Validation in Laravel remains one of its strong suits as we step into 2025. Its expressive rule definitions and rich customization ensure that even the most complex validation requirements can be easily implemented. By mastering validation, you ensure your applications remain secure, efficient, and user-friendly.
Stay updated with Laravel’s future developments and community practices to innovate and improve your applications continually. Happy coding!