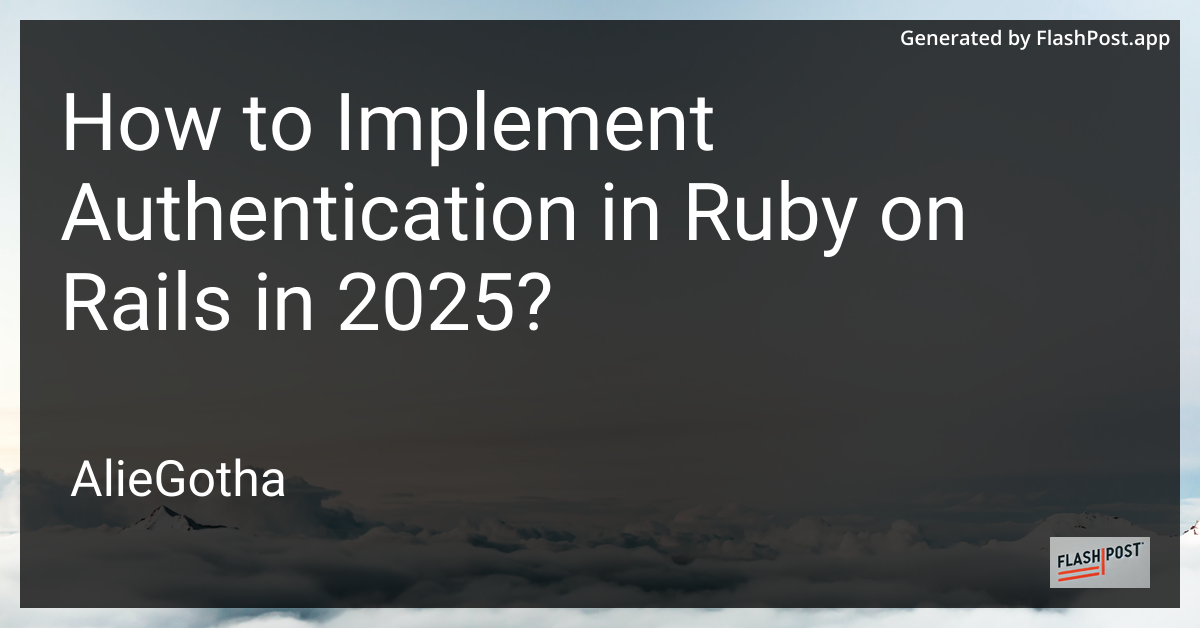
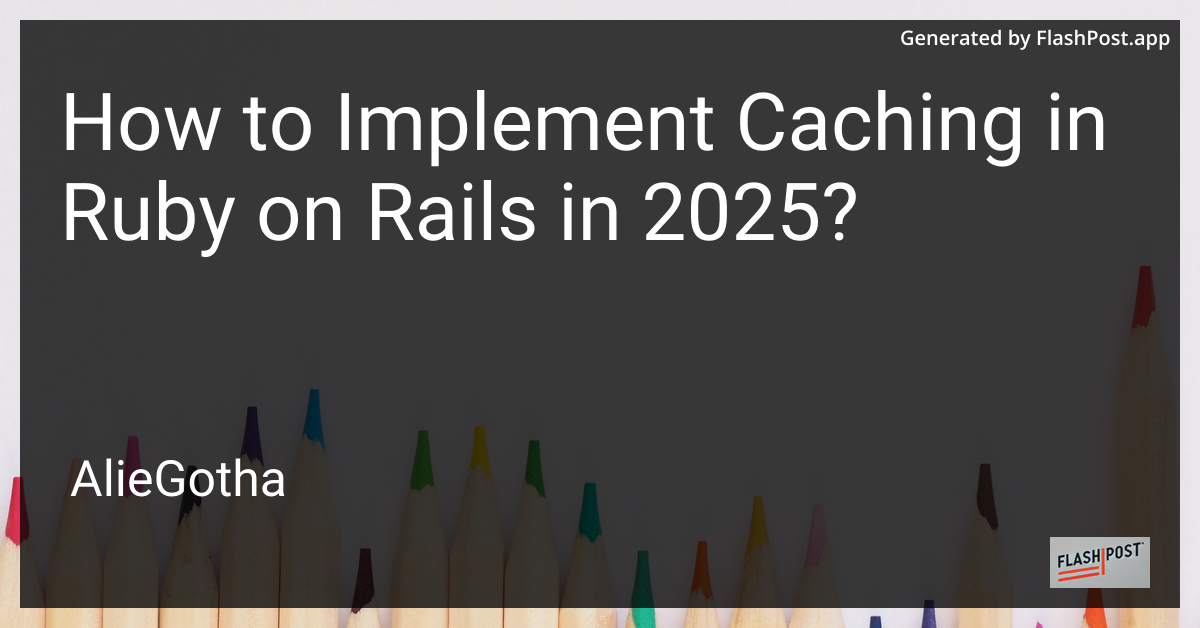
How to Implement Caching in Ruby on Rails in 2025?
As web applications become increasingly complex, performance remains a focal point for developers. One of the most effective ways to improve the performance of your Ruby on Rails application is through caching. This optimization technique stores copies of expensive computations and database queries, effectively reducing load times and server demand. In 2025, leveraging Rails’ caching mechanisms is easier and more robust than ever. This guide will walk you through implementing caching in Ruby on Rails.
Understanding Caching in Ruby on Rails
Caching is a tradeoff between speed and staleness, where you store a computed result and reuse it in subsequent requests. By minimizing database lookups and complex computations, caching can dramatically enhance your app’s performance.
Types of Caching
Rails supports multiple caching methods:
- Page Caching: The simplest form, where entire pages are cached and served without hitting the Rails stack.
- Action Caching: Similar to page caching, but allows for execution of filters before rendering.
- Fragment Caching: Caches fragments of views; useful for pages with dynamic and static parts.
- Low-Level Caching: Such as
Rails.cache
, used to store any Ruby object.
Implementing Caching in Ruby on Rails
Step 1: Choose a Cache Store
First, decide on a cache store. Rails supports file storage, memory storage, Memcached, and Redis as cache stores.
config.cache_store = :mem_cache_store, "cache-1.example.com", "cache-2.example.com"
Step 2: Enable Caching
By default, caching is disabled in the development environment. Activate it by running:
bin/rails dev:cache
Step 3: Implement Page Caching
In 2025, page caching remains straightforward, albeit less common due to dynamic content:
caches_page :index
Step 4: Implement Action Caching
Use action caching when you need authentication checks but want to bypass rendering logic:
caches_action :show
Step 5: Fragment Caching
Use fragment caching to store freestanding parts of a view:
<% cache do %>
<p>Cached content here.</p>
<% end %>
Step 6: Low-Level Caching
For storing calculations or database queries:
Rails.cache.write('today_stats', stats)
stats = Rails.cache.fetch('today_stats')
Step 7: Cache Expiration
Always consider expiration to avoid serving stale data. Set expires_in
or manual sweeps:
Rails.cache.write('expensive_calc', result, expires_in: 12.hours)
Best Practices
- Test Cache Performance: Use tools like MiniProfiler or Rails Panel.
- Monitor Cache Hit Rate: Ensure your cache hit-to-miss ratio justifies the complexity.
- Security Considerations: Never cache sensitive information.
Learning More about Ruby on Rails
Deepen your understanding of Ruby on Rails by exploring related topics:
- How to Build a Forum with Ruby on Rails
- Tips on Ruby on Rails Development
- Comparing Ruby and Ruby on Rails
In conclusion, caching is a powerful tool in your optimization arsenal. By leveraging Rails’ robust caching framework, developers in 2025 can deliver fast and efficient web applications. Embrace these strategies and keep your web applications performing at lightning speed!