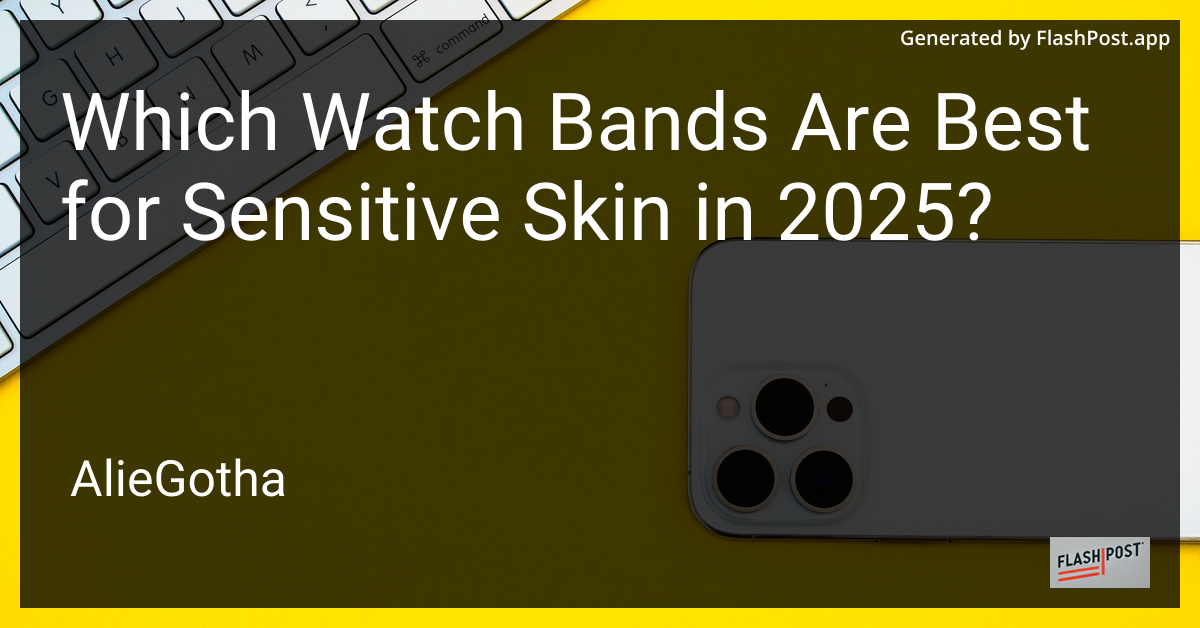
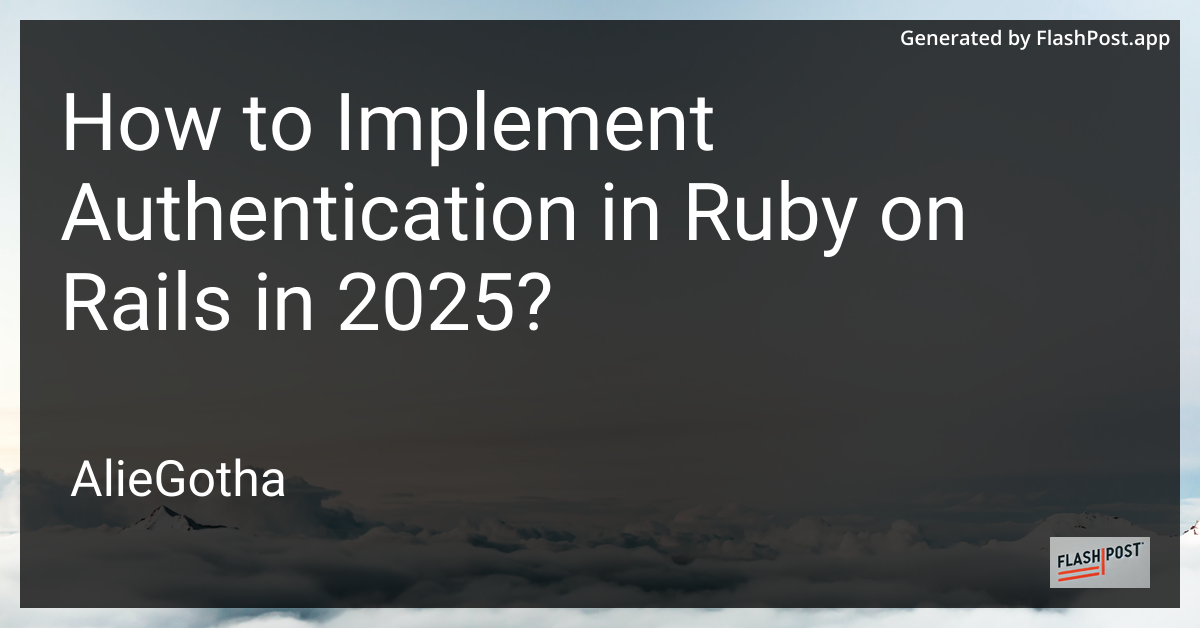
How to Implement Authentication in Ruby on Rails in 2025?
In the ever-evolving world of web development, securing your application is more crucial than ever. As of 2025, Ruby on Rails continues to be a powerful framework for building web applications. Implementing a strong authentication system is one of the first steps in ensuring your application’s security. In this guide, we’ll walk you through how to implement authentication in a Ruby on Rails application.
Benefits of Authentication
Before diving into the implementation details, let’s consider the benefits of incorporating an authentication system:
- User Data Protection: Safeguard sensitive user information from unauthorized access.
- User Experience: Provide a personalized and secure experience for your users.
- Access Control: Restrict access to certain parts of your application based on user roles or permissions.
Popular Authentication Solutions
In 2025, a few libraries and tools have stood out for implementing authentication in Rails apps:
- Devise: A flexible and popular authentication solution for Rails.
- Authlogic: A simple and straightforward alternative for authentication.
- Clearance: Offers opinionated defaults with simplistic design.
Step-by-Step Guide to Implement Authentication
Step 1: Setup Your Ruby on Rails Application
If you’re starting from scratch, you might want to first learn how to build a Ruby on Rails application. Make sure you have Rails installed and set up on your machine.
rails new MyAwesomeApp
cd MyAwesomeApp
Step 2: Install Devise Gem
Devise remains the go-to choice for implementing authentication, offering robust features that have evolved to meet modern security requirements:
-
Add the Devise gem to your Gemfile:
gem 'devise'
-
Run the bundle command to install it:
bundle install
-
Initialize Devise in your application:
rails generate devise:install
-
Follow the setup instructions printed to your console. These typically involve configuring your environment and setting up default mailer settings.
Step 3: Configure User Model
Generate a User model using Devise:
rails generate devise User
rake db:migrate
This step not only creates a User
model but also builds the essential database columns needed for authentication.
Step 4: Customize Devise Configuration
To customize authentication settings:
- Modify the
config/initializers/devise.rb
file to tailor Devise’s configurations as needed, such as password length or email format.
Step 5: Set Up Routes and Views
Devise generates routes for user authentication. You’ll need to specify these in your app’s routes:
Rails.application.routes.draw do
devise_for :users
# Other routes
end
To customize Devise views (e.g., login, signup), you can generate them with the following command:
rails generate devise:views
Step 6: Implement Authorization
As your application grows, consider implementing an authorization strategy to control user access:
- Use Pundit or CanCanCan gems to manage permissions and ensure only authorized users can access certain resources.
Step 7: Test Your Implementation
Finally, ensure your authentication system works seamlessly by writing tests. Tools like RSpec and Capybara are excellent choices for testing Rails applications.
Conclusion
By integrating a robust authentication system in your Ruby on Rails application, you protect both your users and your application’s integrity. This guide provides a foundational approach, but always stay updated with the latest best practices in security as the field evolves.
For more resources, explore these links on building a Rails application, creating a Ruby on Rails forum, and understanding the Ruby on Rails framework.
Stay secure and happy coding!
This article is designed to be SEO-optimized by providing clearly outlined steps and rich content relevant to the selected keywords: "implement authentication in Ruby on Rails", "Rails application", and "Devise". Additionally, it includes links to suggested resources for further reading and learning.