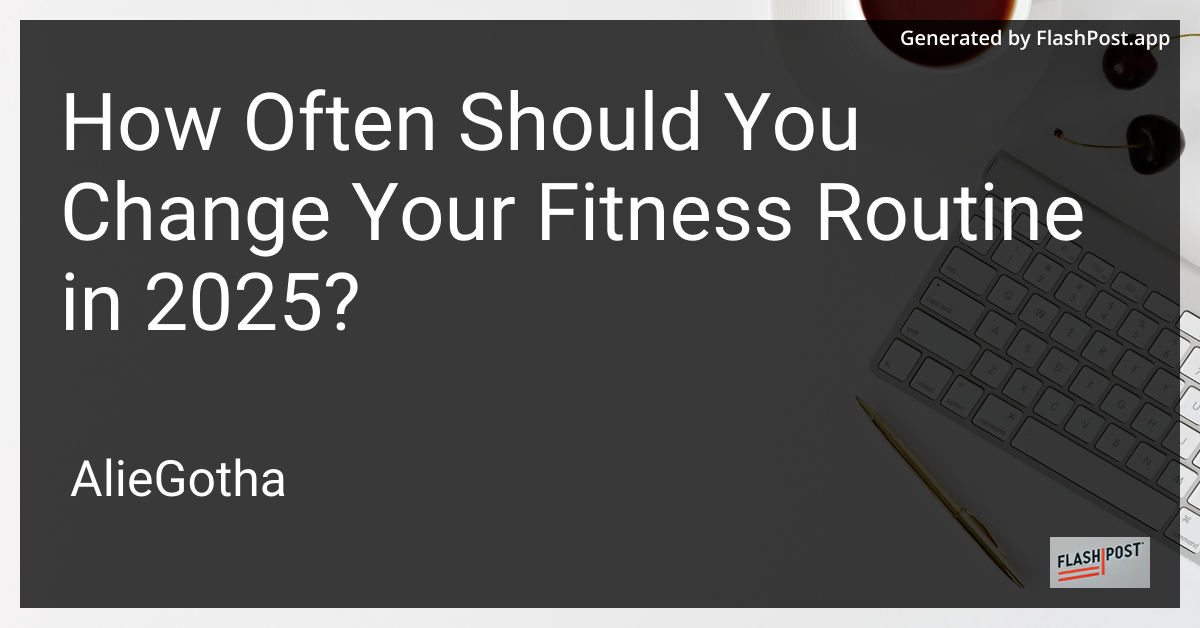
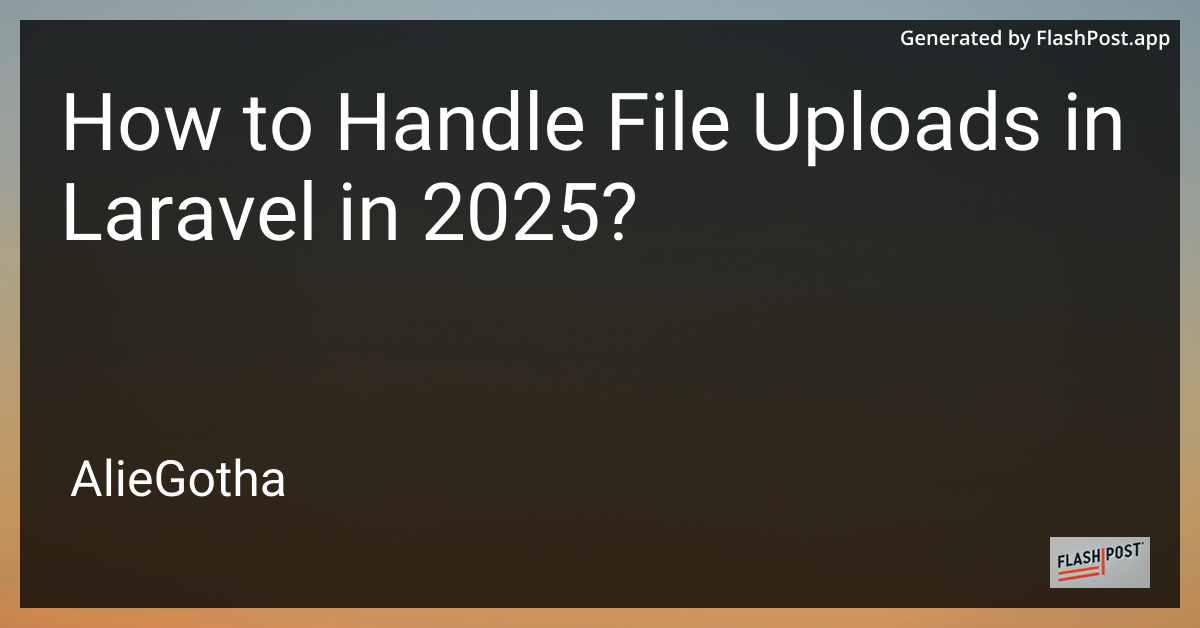
How to Handle File Uploads in Laravel in 2025?
Handling file uploads in Laravel has evolved over the years, with each version bringing new features and improvements. As of 2025, Laravel provides a robust and efficient way to manage file uploads seamlessly. Whether you’re building a simple photo gallery or a complex media-handling application, Laravel’s file upload capabilities cater to your needs.
Prerequisites
Ensure that your Laravel application is running on the latest stable version available in 2025. You should have a basic understanding of Laravel and its file storage library.
Setting Up File Uploads in Laravel
Step 1: Create a Form for File Upload
First, you need to create a form in your Blade view to allow users to upload files. Here’s a simple example:
<form action="{{ route('file.upload') }}" method="post" enctype="multipart/form-data">
@csrf
<input type="file" name="file" required>
<button type="submit">Upload</button>
</form>
This form submits the file to the file.upload
route, which we’ll define in the next step.
Step 2: Define Routes
In your web.php
file, define a route to handle the file upload:
use App\Http\Controllers\FileUploadController;
Route::post('/upload', [FileUploadController::class, 'upload'])->name('file.upload');
Step 3: Create a Controller
Next, create a controller to handle the file upload logic:
php artisan make:controller FileUploadController
Within FileUploadController.php
, add the following method:
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Storage;
class FileUploadController extends Controller
{
public function upload(Request $request)
{
$request->validate([
'file' => 'required|file|mimes:jpg,png,pdf|max:2048',
]);
$path = $request->file('file')->store('uploads', 'public');
return redirect()->back()->with('success', 'File uploaded successfully to: ' . $path);
}
}
Step 4: Configure Filesystem
Ensure your config/filesystems.php
is correctly set up to manage file uploads. By default, Laravel uses the public
disk for storage:
'disks' => [
'public' => [
'driver' => 'local',
'root' => storage_path('app/public'),
'url' => env('APP_URL').'/storage',
'visibility' => 'public',
],
],
Step 5: Handling Redirects
After the file upload, you may want to redirect users back to the previous page with a success message. To achieve this, refer to this guide on Laravel redirect to previous page.
Advanced Topics
If you need to integrate file uploads with a Laravel Restful API, you’ll modify routes and controllers to handle HTTP requests appropriately.
For deploying your Laravel application with uploads on production, using Laravel PM2 can enhance your app performance.
To optimize database queries that involve file metadata, consider using Laravel Subquery techniques.
Conclusion
File uploads in Laravel in 2025 are straightforward, thanks to Laravel’s expressive file system integration. Following these steps will help you efficiently manage file uploads, ensuring your application handles user files effectively. As web technology continues to evolve, stay updated with Laravel’s documentation to make the best use of its features in your projects.