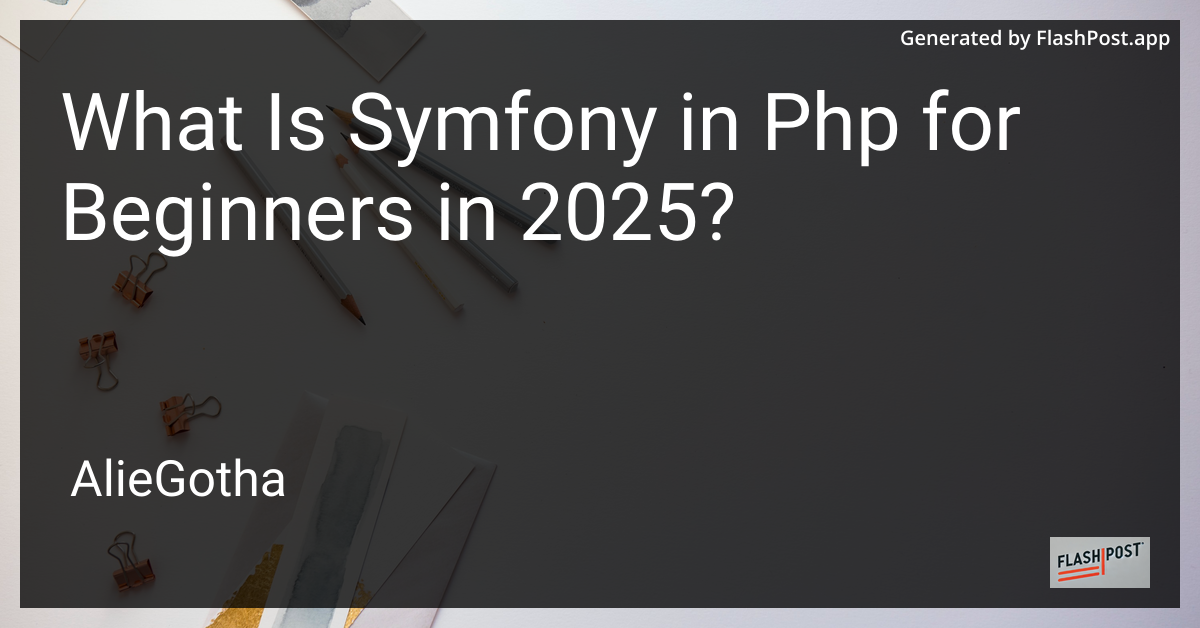
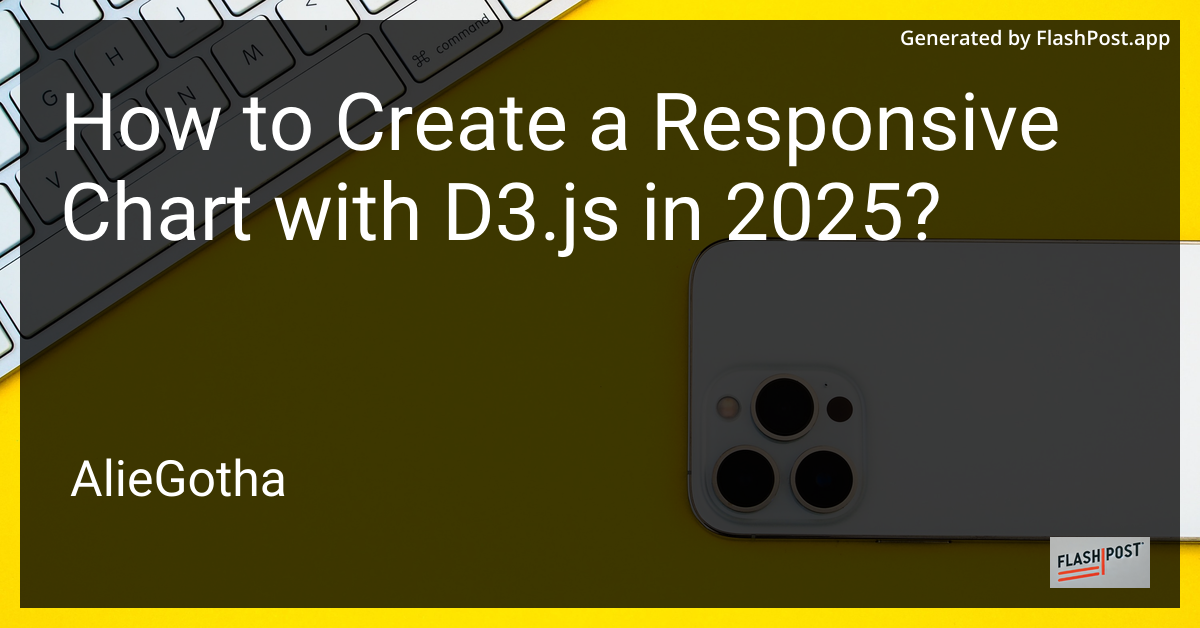
How to Create a Responsive Chart with D3.js in 2025?
In the world of data visualization, D3.js stands out as one of the most powerful libraries available. As the web evolves, creating responsive charts that adapt to various screen sizes is crucial. By 2025, mastering responsive design in D3.js will not only enhance user experience but also improve accessibility and engagement with your data visualizations. In this guide, we’ll explore how to create a responsive chart with D3.js, ensuring that your data looks great on any device.
Understanding Responsive Design in D3.js
Responsive design allows your chart to adjust automatically to different screen sizes and orientations. This is critical in modern web development, where users access content from a range of devices like smartphones, tablets, and desktops. To facilitate responsiveness in D3.js, consider the following steps:
-
Set SVG Dimensions Proportionally: Use relative units or percentages for your SVG container instead of fixed dimensions. This helps the SVG element adjust as the parent container resizes.
-
Scale Axes and Elements: Utilize D3.js scales and axis functions to dynamically adjust your chart elements based on the SVG’s current size. This ensures consistency across different resolutions.
-
Flexible Margin Convention: Adopt a flexible margin convention where the margins adjust proportionally to the SVG size. This maintains the integrity of your chart structure across various screen sizes.
Step-by-Step Guide to Creating a Responsive Chart
Step 1: Set Up Your Environment
Ensure you have D3.js integrated into your project by adding the latest version of the library. You can include it via a CDN or install it through npm if you’re using a build tool.
<script src="https://d3js.org/d3.v7.min.js"></script>
Step 2: Create a Flexible SVG Container
Define an SVG element with a viewBox attribute. This helps in scaling the SVG content while maintaining the aspect ratio.
const svg = d3.select("body").append("svg")
.attr("viewBox", "0 0 1000 500")
.attr("preserveAspectRatio", "xMinYMin meet")
.style("width", "100%")
.style("height", "auto");
Step 3: Design Your Chart
Depending on your data, design your chart elements like bars, lines, or pie sections. Use D3.js’s powerful data binding capabilities to draw these elements.
Step 4: Responsive Scaling
Implement responsive scales that recalculate whenever the window resizes. Utilize D3.js’s scaleLinear
or scaleBand
for dynamic scaling.
const xScale = d3.scaleLinear()
.domain([0, d3.max(data)])
.range([0, parseFloat(svg.style('width'))]);
Step 5: Listen for Resize Events
Add an event listener to adjust your chart when the window resizes.
window.addEventListener('resize', () => {
const newWidth = parseFloat(svg.style('width'));
xScale.range([0, newWidth]);
svg.selectAll("rect")
.attr("x", (d) => xScale(d));
});
Explore More about D3.js
- Learn how to reduce SVG size: D3.js Development
- Add legends to your charts: D3.js Legend
- Add interactive elements like bubble charts: D3.js Bubble Chart
Conclusion
Creating responsive charts in D3.js can significantly enhance your data visualization projects. By following this guide and integrating the suggested techniques, you’ll ensure your charts are adaptable, user-friendly, and visually appealing on all devices. Embrace these practices in 2025 and stay ahead in the ever-evolving world of web development and data visualization.