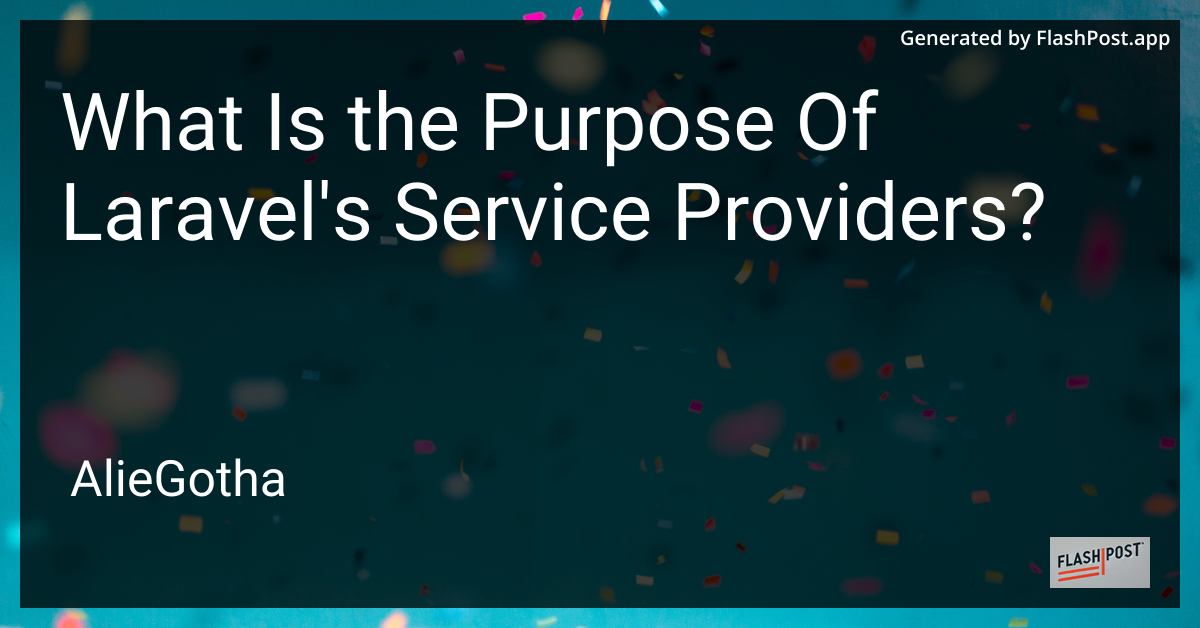
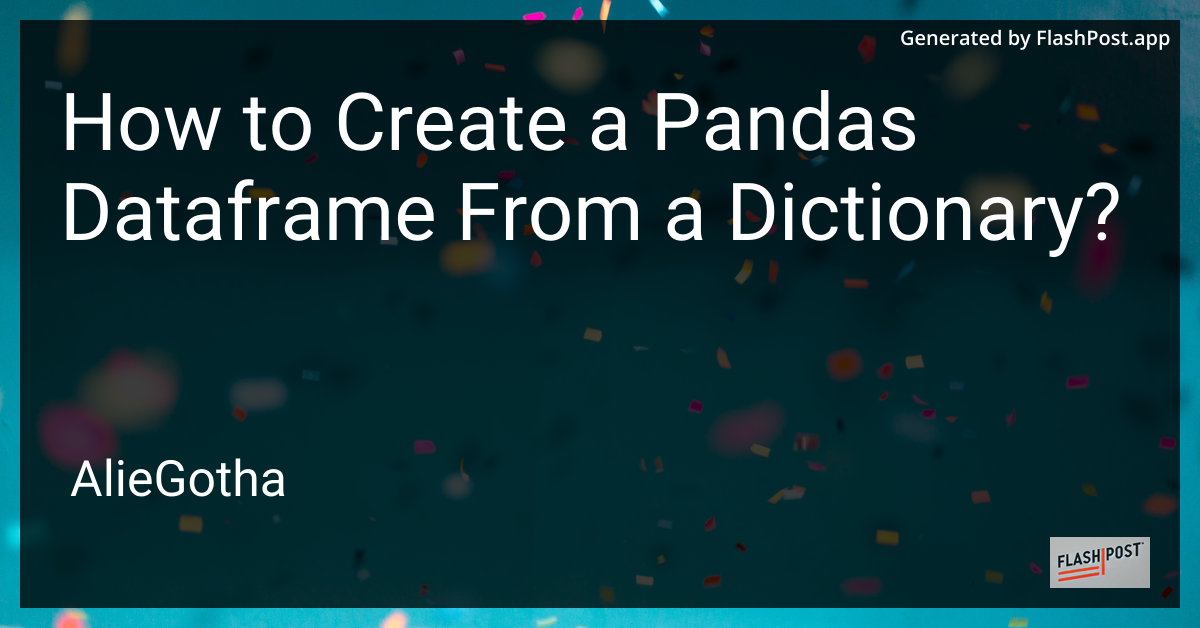
How to Create a Pandas Dataframe From a Dictionary?
Pandas is a powerful and widely used data manipulation library in Python. One of the most common tasks in data analysis and manipulation is creating a Pandas DataFrame. If you’re working with Python, you’ll often need to create a DataFrame from standard Python data structures, such as dictionaries. Here, we’ll guide you through creating a Pandas DataFrame from a dictionary, a fundamental skill for any data scientist or analyst working with Python.
Creating a Pandas DataFrame
Creating a Pandas DataFrame from a dictionary is straightforward, and it can be accomplished in a few simple steps. First, you’ll need to have Pandas installed in your Python environment. If you haven’t done this yet, you can install it using pip:
pip install pandas
Once you’ve ensured Pandas is installed, you can proceed with creating a DataFrame from a dictionary.
Step-by-Step Guide
-
Import Pandas: First, you’ll need to import the Pandas library.
import pandas as pd
-
Prepare Your Dictionary: Create a dictionary where the keys will be the column names and the values will be the data for those columns.
data = { 'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35], 'City': ['New York', 'Los Angeles', 'Chicago'] }
-
Create the DataFrame: Use the
pd.DataFrame
function to create the DataFrame from your dictionary.df = pd.DataFrame(data)
-
View the DataFrame: Print the DataFrame to see how it looks.
print(df)
Example Code
Here’s a complete example of how you can do this in one go:
import pandas as pd
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'Los Angeles', 'Chicago']
}
df = pd.DataFrame(data)
print(df)
Output:
Name Age City
0 Alice 25 New York
1 Bob 30 Los Angeles
2 Charlie 35 Chicago
Additional Resources
Creating Pandas DataFrames is just the beginning. Here are some resources for further exploration on working with DataFrames:
- Learn to plot your Pandas DataFrame using SymPy.
- Discover how to conduct a Pandas DataFrame comparison.
- Explore how to convert DataFrame columns into JSON.
- Find out how to add rows to a DataFrame in Pandas.
- Learn about Pandas DataFrame manipulation.
Conclusion
Creating a Pandas DataFrame from a dictionary is an essential skill for anyone working with data in Python. It allows you to quickly and easily transform a simple Python data structure into a powerful tool for data manipulation and analysis. By understanding how to create DataFrames, you’re opening the door to the extensive capabilities of the Pandas library. For more complex operations and data manipulations, explore the additional resources linked above. Happy coding!