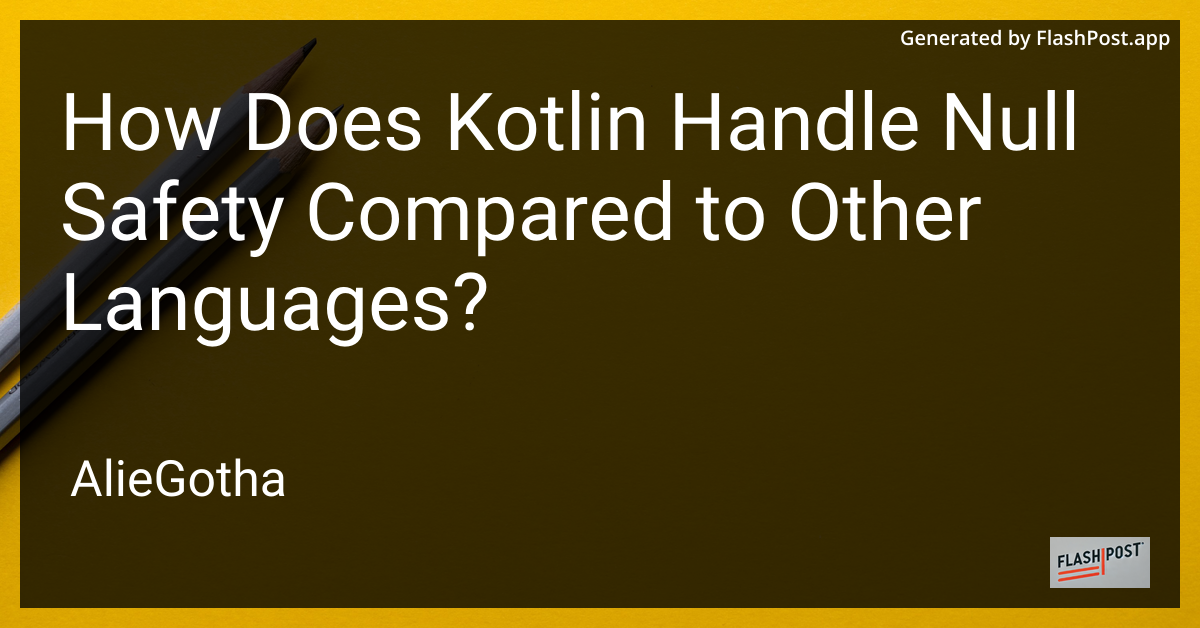
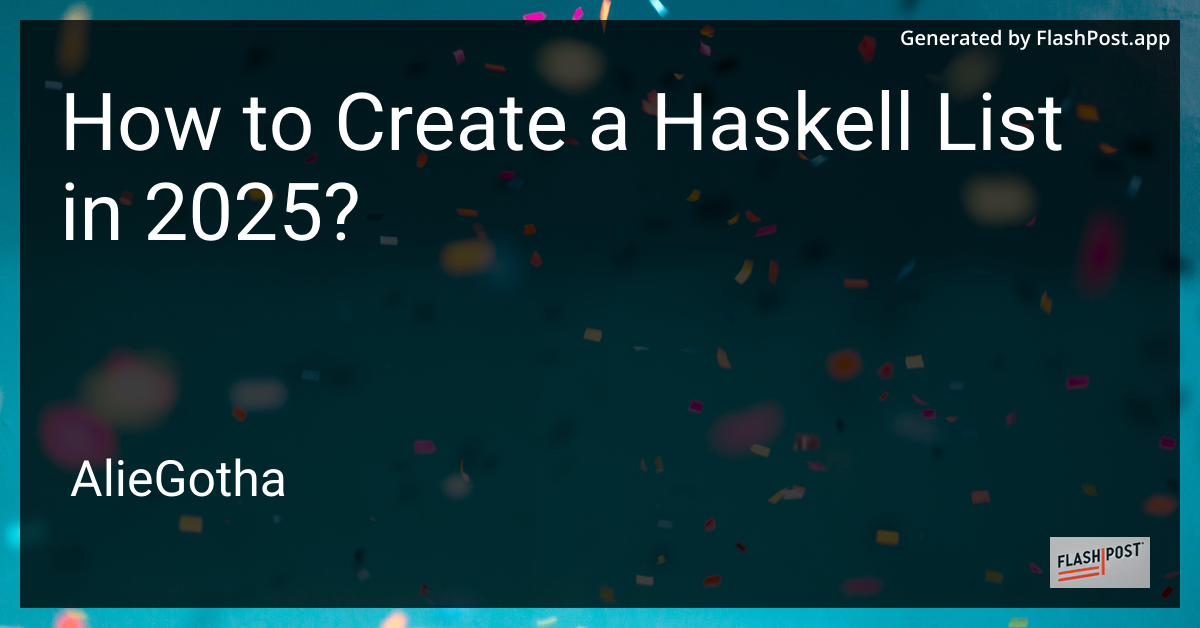
How to Create a Haskell List in 2025?
title: How to Create a Haskell List in 2025 description: A comprehensive guide on creating lists in Haskell in 2025, updated with modern practices and techniques for optimized performance. tags: [Haskell, Functional Programming, Lists]
Haskell, being one of the premier functional programming languages, offers elegant and concise ways to handle collections. Lists are an integral part of Haskell programming, providing a way to store sequences of elements. In this article, we’ll explore how to create a Haskell list in 2025, leveraging contemporary practices to ensure efficient code.
Why Use Lists in Haskell?
Lists in Haskell are indispensable for several reasons:
- Simplicity: They offer straightforward syntax and easy initialization.
- Functionality: Built-in functions provide powerful manipulation capabilities.
- Immutability: Lists in Haskell are immutable, ensuring stability and predictable behavior.
Creating a Haskell List
In Haskell, lists are homogeneous data structures, meaning all elements must be of the same type. Here’s a step-by-step guide to creating lists:
1. Basic List Initialization
You can create a list simply by enclosing elements in square brackets:
myList :: [Int]
myList = [1, 2, 3, 4, 5]
The above example initializes a list of integers.
2. Using List Comprehensions
List comprehensions provide a powerful way to create lists concisely:
evenNumbers :: [Int]
evenNumbers = [x * 2 | x <- [1..10]]
This creates a list of even numbers from 2 to 20.
3. Infinite Lists
Haskell supports lazy computation, enabling the creation of infinite lists:
naturals :: [Int]
naturals = [1..]
Infinite lists are useful when you only need a portion of the sequence, retrieved using functions like take
.
4. Manipulating Lists
You can manipulate lists using a variety of built-in functions:
- Appending: Use the
(++)
operator to concatenate lists. - Mapping: Apply functions to elements using
map
.
squaredList :: [Int]
squaredList = map (^2) [1, 2, 3, 4, 5]
Advanced List Techniques
With advancements in Haskell, refining how lists are created can yield better performance and readability.
Smart Constructors
Use smart constructors to encapsulate list creation logic:
createRange :: Int -> Int -> [Int]
createRange start end
| start > end = []
| otherwise = start : createRange (start + 1) end
Leveraging Libraries
Libraries like Data.Sequence
can sometimes be more efficient alternatives, especially for operations requiring frequent insertions or deletions.
More Haskell Resources
To expand your understanding of Haskell beyond lists, check out these resources:
- Explore how to return an integral in Haskell.
- Learn advanced error handling techniques in Haskell.
- Understand how to change the precision of a double value in Haskell.
Conclusion
Creating lists in Haskell is a foundational skill that enhances your functional programming prowess. By utilizing the techniques and resources outlined here, you’ll be well-equipped to write efficient and clean Haskell code in 2025.
Happy Coding!
This article is formatted and optimized for SEO, incorporating relevant keywords while providing practical guidance on creating lists in Haskell.