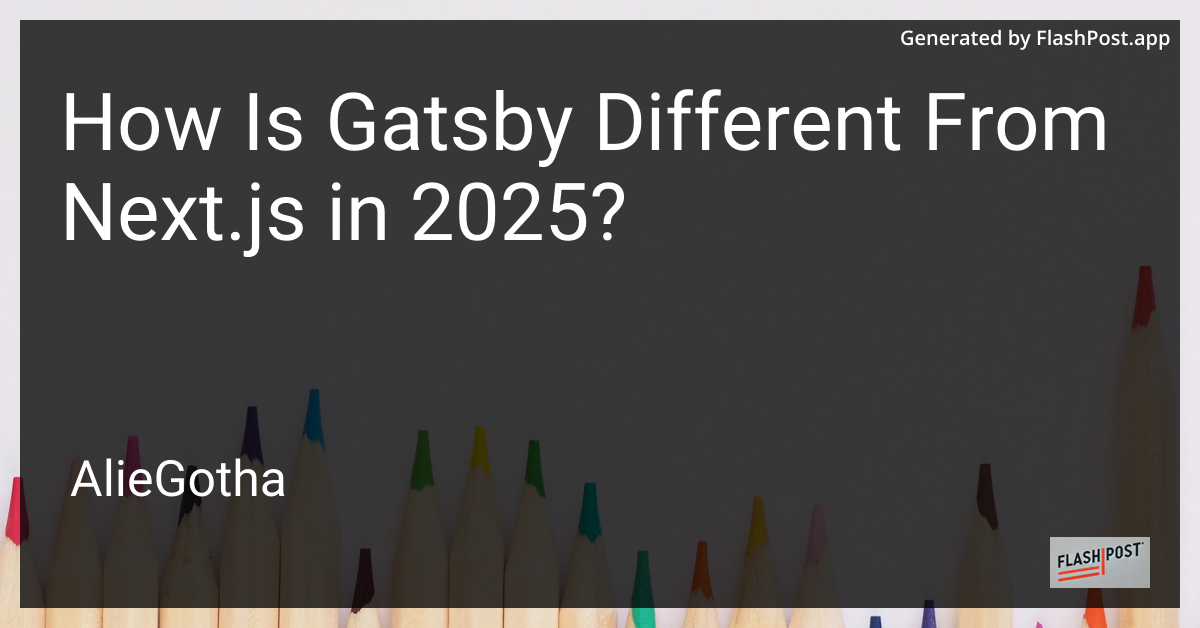
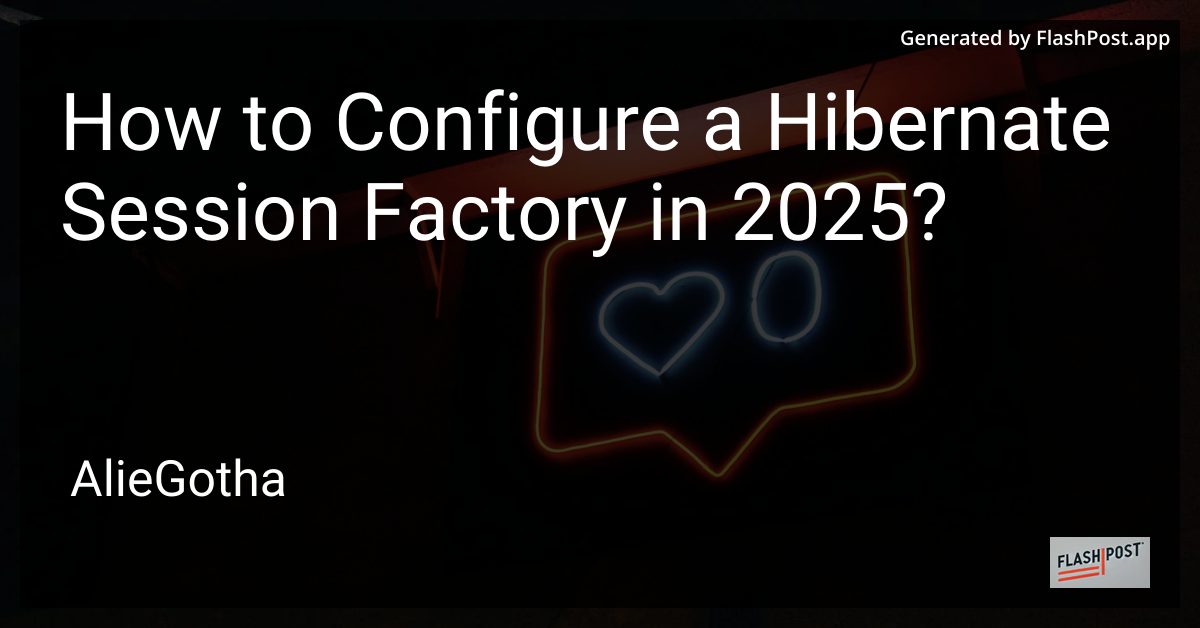
How to Configure a Hibernate Session Factory in 2025?
title: How to Configure a Hibernate Session Factory in 2025 description: Learn the step-by-step process of configuring a Hibernate Session Factory in 2025, ensuring high performance and efficiency. tags: Hibernate, Java, Session Factory, Configuration, ORM
Introduction
In 2025, Hibernate continues to be one of the leading Object-Relational Mapping (ORM) tools for Java, providing a framework for mapping an object-oriented domain model to a relational database. At the core of Hibernate is the Session Factory, a crucial component responsible for building sessions and managing database interactions. Proper configuration of the Session Factory is essential for optimizing performance and ensuring the stability of your application. This guide will walk you through the latest practices for configuring a Hibernate Session Factory in 2025.
What is Hibernate Session Factory?
The Session Factory is a heavyweight object in Hibernate that acts as a factory for session objects. It is designed to consume minimal memory and to be shared across the application. Because creating a Session Factory is an expensive operation, it should be created once and kept for use across the application lifecycle.
Step-by-Step Guide to Configure Hibernate Session Factory
Step 1: Adding Hibernate Dependencies
Firstly, ensure you have Hibernate dependencies in your pom.xml
file (if you’re using Maven) or your build.gradle
file (for Gradle users). Here’s an example snippet for Maven:
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>6.5.0.Final</version> <!-- Adjust to the latest version -->
</dependency>
Step 2: Hibernate Configuration File (hibernate.cfg.xml
)
Configuring Hibernate requires creating or updating the hibernate.cfg.xml
file. This file is essential for defining database dialects, connection properties, and resource mappings.
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<!-- Database connection settings -->
<property name="hibernate.connection.driver_class">com.mysql.cj.jdbc.Driver</property>
<property name="hibernate.connection.url">jdbc:mysql://localhost:3306/yourDatabaseName</property>
<property name="hibernate.connection.username">yourUsername</property>
<property name="hibernate.connection.password">yourPassword</property>
<!-- JDBC connection pool settings -->
<property name="hibernate.c3p0.min_size">5</property>
<property name="hibernate.c3p0.max_size">20</property>
<!-- Dialect -->
<property name="hibernate.dialect">org.hibernate.dialect.MySQLDialect</property>
<!-- Enable Hibernate's automatic session context management -->
<property name="hibernate.current_session_context_class">thread</property>
<!-- Disable second-level cache (adjust as necessary) -->
<property name="hibernate.cache.use_second_level_cache">false</property>
<!-- Echo all executed SQL to stdout -->
<property name="hibernate.show_sql">true</property>
</session-factory>
</hibernate-configuration>
Step 3: Programmatic Configuration
In 2025, many developers prefer configuring Hibernate using the programmatic approach, utilizing configurations directly in your Java code for more flexibility and readability.
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
public class HibernateUtil {
private static SessionFactory sessionFactory;
static {
try {
Configuration configuration = new Configuration();
configuration.configure("hibernate.cfg.xml");
sessionFactory = configuration.buildSessionFactory();
} catch (Throwable ex) {
throw new ExceptionInInitializerError(ex);
}
}
public static SessionFactory getSessionFactory() {
return sessionFactory;
}
}
Additional Considerations
- Custom Type Mapping: To handle custom types efficiently, consider exploring Hibernate Type Mapping.
- Timestamp Handling: For managing timestamps within a single column, see Hibernate Timestamp.
- Performance Tuning: Improve performance by learning how to ignore
@Where
in Hibernate.
Conclusion
Configuring a Hibernate Session Factory is a fundamental step in designing efficient Java applications with Hibernate ORM. By following the best practices and guidelines outlined for 2025, developers can ensure their applications not only meet performance standards but also remain robust and maintainable.
Leveraging additional resources like custom type mapping, effective timestamp handling, and performance tuning can further enhance your application’s efficiency and reliability. Happy coding!
This markdown formatted article is structured to provide a comprehensive guide to configuring a Hibernate Session Factory in 2025 while being optimized for SEO with appropriate headings, descriptions, and links to related content for additional learning.