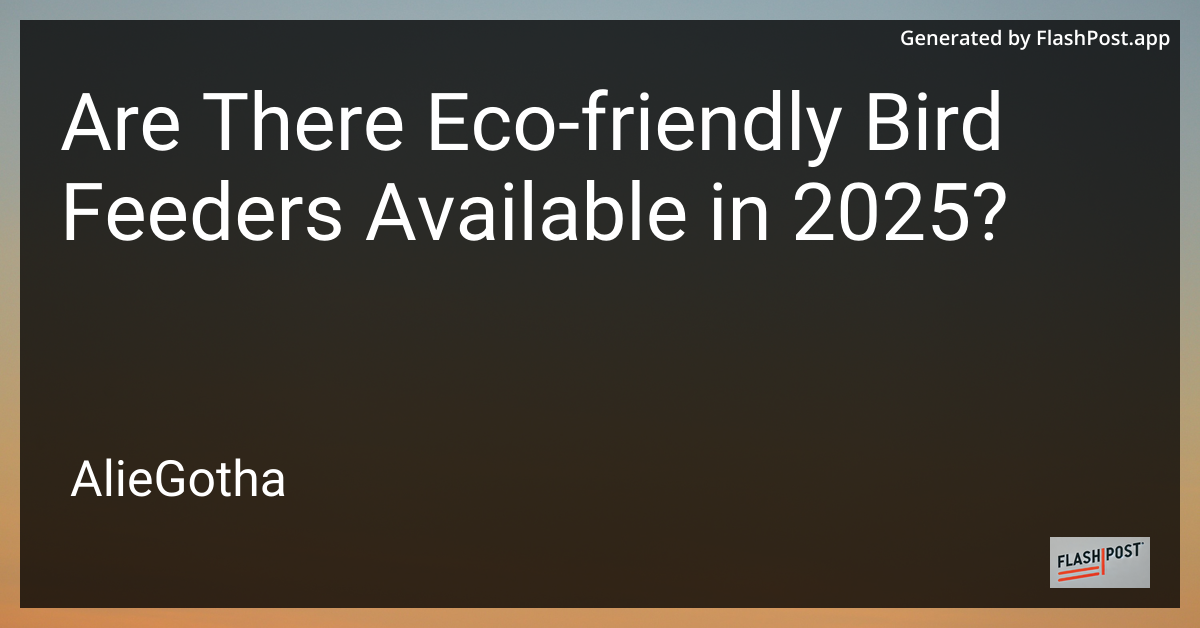
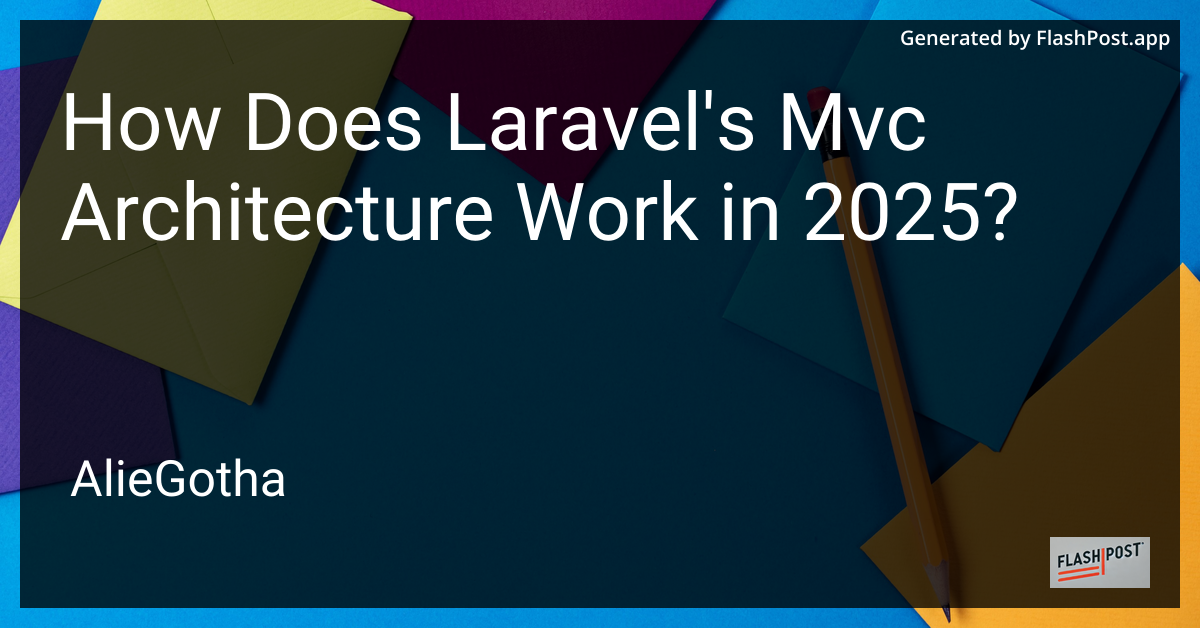
How Does Laravel's Mvc Architecture Work in 2025?
In the world of web development, frameworks are constantly evolving to meet new demands and improve developer productivity. One of the most prominent PHP frameworks is Laravel, which still relies on the MVC (Model-View-Controller) architecture. In 2025, Laravel continues to refine and enhance its MVC implementation, making it a robust tool for developers. This article explores how Laravel’s MVC architecture works in 2025, providing insights into its components and workflow.
Understanding MVC in Laravel
MVC is a software architectural pattern that separates the application into three interconnected components: Model, View, and Controller. This separation helps manage complex applications by organizing code based on its function, improving maintainability, scalability, and testability.
1. Model
The Model represents the application’s data structure. In Laravel, models are primarily responsible for interacting with the database. They define the data properties and relationships, implement business logic, and provide a clean API for database operations.
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class Article extends Model {
// Define properties and relationships
protected $fillable = ['title', 'content', 'author_id'];
}
2. View
The View is responsible for the presentation layer of the application. In Laravel, views are typically written using the Blade templating engine, which allows developers to create dynamic and reusable HTML templates with ease. Views receive data from the Controller and render the user interface.
<!-- resources/views/article.blade.php -->
<h1>{{ $article->title }}</h1>
<p>{{ $article->content }}</p>
<p>Author: {{ $article->author->name }}</p>
3. Controller
Controllers act as an intermediary between Models and Views. They handle user requests, retrieve data from Models, and pass it to Views. In Laravel, controllers define the application logic and manage the flow of data.
namespace App\Http\Controllers;
use App\Models\Article;
use Illuminate\Http\Request;
class ArticleController extends Controller {
public function show($id) {
$article = Article::find($id);
return view('article', compact('article'));
}
}
The MVC Workflow in Laravel
In 2025, despite innovations and improvements in technology, Laravel’s MVC workflow remains fundamentally the same:
- User Interaction: A user interacts with the application’s interface, such as by clicking a link or submitting a form.
- Routing: Laravel’s router receives the request and directs it to the appropriate Controller.
- Controller Logic: The Controller processes the request, retrieves or manipulates data using the Model, and determines the View to be rendered.
- View Rendering: The View is rendered with the data provided by the Controller, generating an HTML response for the user.
Enhancements in 2025
In 2025, Laravel continues to optimize its MVC pattern with the following enhancements:
- Improved Eloquent: Laravel’s ORM, Eloquent, enhances Model capabilities with more efficient querying and relationship handling.
- Blade Components: The Blade templating engine introduces new components and slots features, allowing more modular and maintainable views.
- Middleware Enhancements: Middleware becomes more powerful, providing better tools for managing HTTP requests and responses.
Additional Resources
For specialized topics on Laravel, you may find the following resources helpful:
- Learn how to fetch data from a JSON file with Laravel.
- Discover how to display PDF files using an iframe in Laravel.
- Find out how to prevent Laravel from caching files.
By understanding Laravel’s MVC architecture in 2025, developers can continue to build efficient, organized, and powerful web applications, harnessing the latest features and enhancements offered by Laravel.