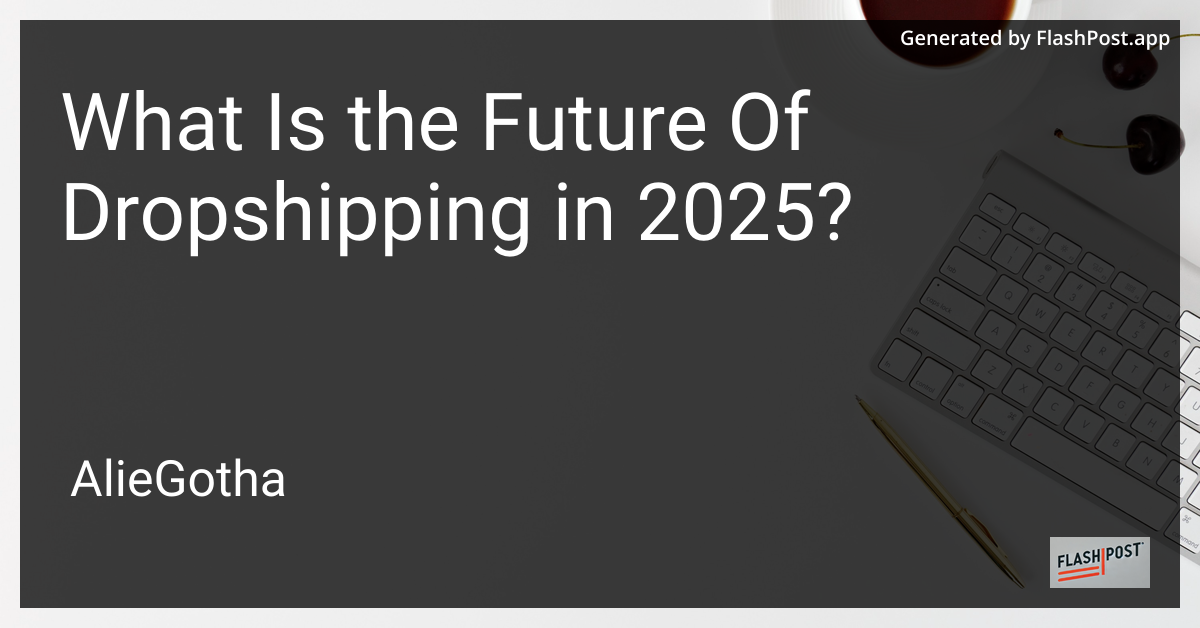
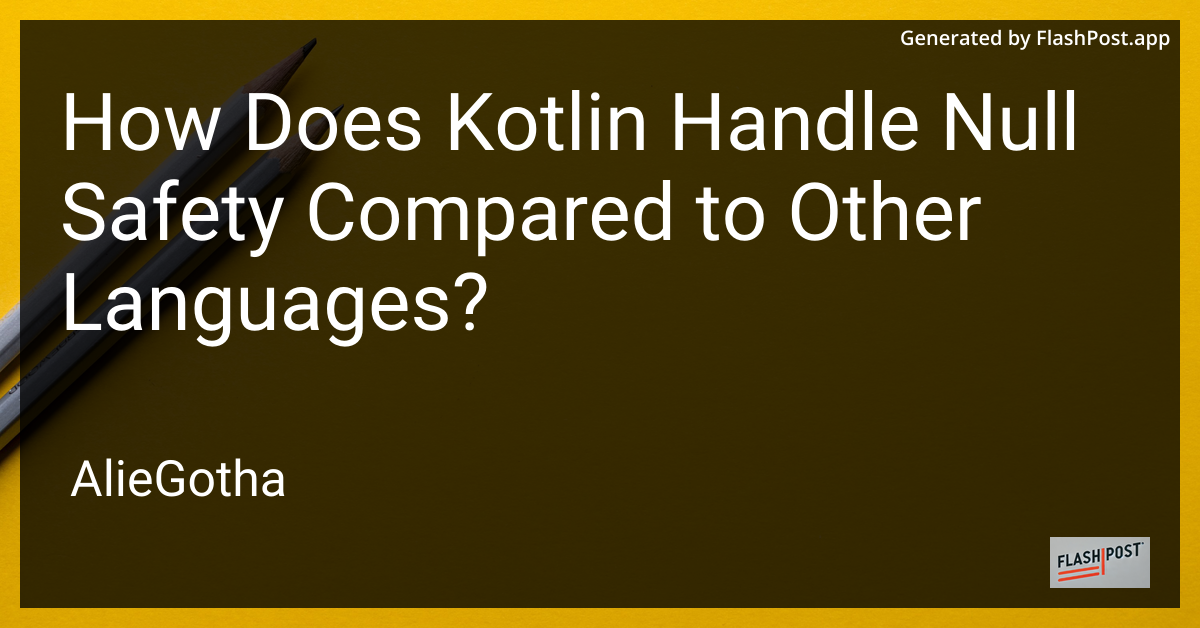
How Does Kotlin Handle Null Safety Compared to Other Languages?
In the landscape of modern programming, dealing with null references is a common source of bugs and errors. Kotlin, the language developed by JetBrains, offers a robust solution to this perennial problem with its emphasis on null safety. In this article, we’ll delve into Kotlin’s approach to handling null safety and compare it to other programming languages.
Understanding Null Safety in Programming
Nullability refers to references that might be absent, resulting in potential runtime errors commonly known as null pointer exceptions. Many languages treat null as a potential state for every reference unless explicitly stated otherwise. However, Kotlin takes a more proactive stance against null-related issues.
Kotlin’s Approach to Null Safety
Kotlin integrates null safety directly into its type system, thereby reducing bugs from null references:
-
Nullable and Non-Nullable Types: By default, all types in Kotlin are non-nullable. If you want a variable to hold a null, you have to declare it with a nullable type, signified by a
?
. For example:var nullableVar: String? = null var nonNullableVar: String = "Hello"
-
Safe Calls and the Elvis Operator: Kotlin employs safe calls (
?.
) to safely access properties and methods of nullable types. If the reference is null, the method call returns null without throwing an exception. Additionally, the Elvis operator (?:
) provides a default value in case the preceding expression is null:val length = nullableVar?.length ?: 0
-
Not-Null Assertions: Kotlin introduces the not-null assertion operator (
!!
) to explicitly throw an exception if a value is null. This should be used cautiously:val nonNullLength = nullableVar!!.length
Comparing Null Safety in Other Languages
Java
Java traditionally treats null references as a potential state for all objects, which results in the infamous NullPointerException
when attempting to access methods or properties on a null reference. To handle nullability, Java 8 introduced Optional, but its verbosity and lack of enforcement make it less intuitive than Kotlin’s approach.
Swift
Swift, like Kotlin, integrates null safety into its type system with Optionals, denoted using ?
. It uses optional chaining (similar to Kotlin’s safe calls) and provides if let
or guard let
for safely unwrapping optionals, which is conceptually similar to Kotlin’s null checks.
C#
C# introduced nullable value types and nullable reference types in later versions, allowing developers to explicitly specify nullability. Like Kotlin, it uses the ?
syntax but lacks the same level of seamless integration with the type system, affecting ease of use.
Why Kotlin Stands Out
Kotlin’s null safety features are seamlessly integrated into the language, easing development and reducing runtime errors. Its innovation in treating nullability as an explicit choice provides a more disciplined, less error-prone environment which is especially useful in Android development and web applications.
For further reading on Kotlin programming techniques and web development with Kotlin, explore these resources:
Kotlin sets a benchmark for null safety, empowering developers with an intuitive, null-safe coding experience that enhances productivity and software reliability.
In conclusion, Kotlin’s robust handling of null safety distinguishes it from many other modern programming languages. By integrating stringent null-safety checks, Kotlin minimizes the likelihood of null-related runtime errors, establishing itself as a preferred language for developing reliable and maintainable applications.