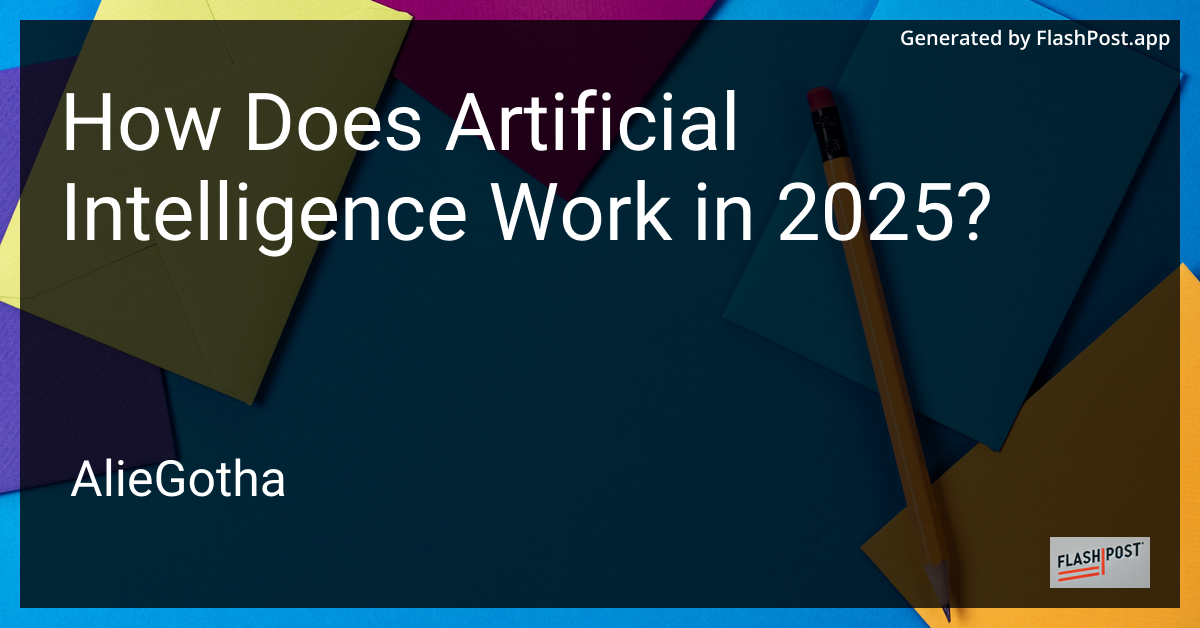
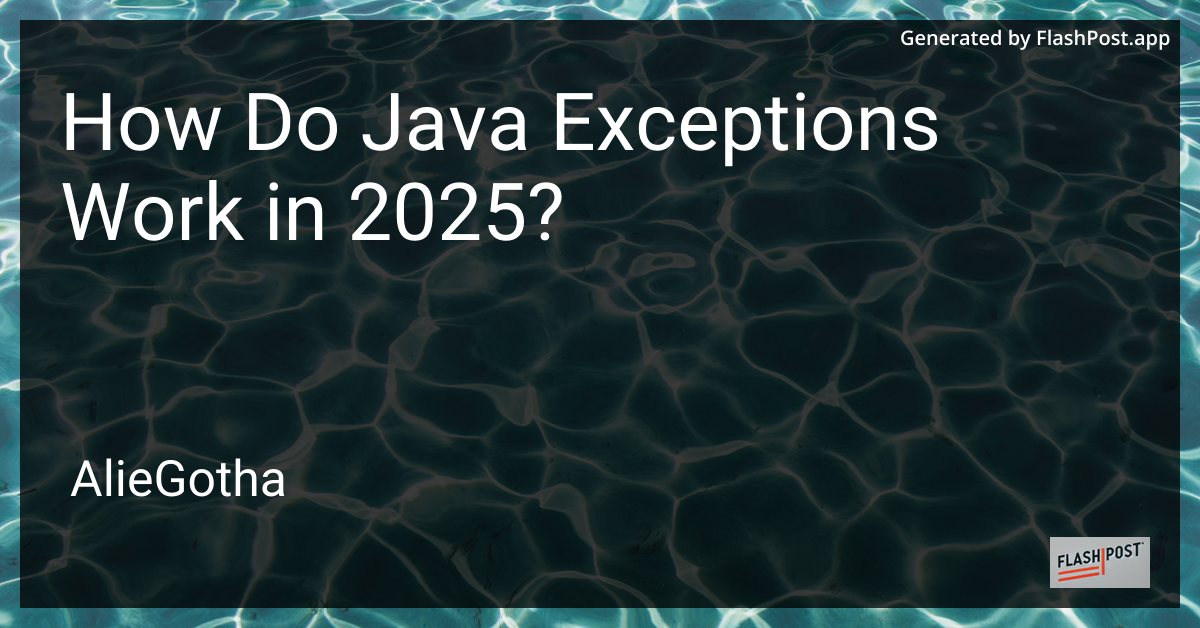
How Do Java Exceptions Work in 2025?
In the world of programming, the ability to manage errors gracefully is vital. Java, a widely-used programming language, provides a robust mechanism for handling errors through exceptions. In this guide, we’ll explore how Java exceptions work, especially as of 2025, and how they help maintain seamless application flow.
What are Java Exceptions?
Exceptions in Java are events that occur during the execution of a program that disrupts the normal flow of instructions. When a method encounters an issue it cannot handle, it throws an exception. This allows the issue to be caught and managed, preventing the program from crashing and allowing a smooth user experience.
Types of Exceptions
Java exceptions are primarily categorized into three types:
-
Checked Exceptions: These are the exceptions checked at compile time. They must be handled using a try-catch block or declared in the method signature using the
throws
keyword. For example,IOException
. -
Unchecked Exceptions: These include Runtime Exceptions and are not checked at compile time. They can occur from programming errors, such as logic errors or improper use of an API. An example is
NullPointerException
. -
Errors: These are severe problems that the application should not attempt to handle. Errors are not exceptions but are represented as a subclass of Throwable. Examples include
OutOfMemoryError
.
How Java Exception Handling Works
Java exception handling is implemented using five keywords: try
, catch
, finally
, throw
, and throws
.
-
try: A block of code that might throw an exception.
-
catch: A block that handles the exception thrown by the try block.
-
finally: An optional block that executes after try-catch, irrespective of whether an exception is thrown or not.
-
throw: Used to explicitly throw an exception.
-
throws: Used in the method signature to declare the exceptions that can be thrown by the method.
Example of Exception Handling
public class ExceptionExample {
public static void main(String[] args) {
try {
int division = 10 / 0; // This will throw ArithmeticException
} catch (ArithmeticException e) {
System.out.println("ArithmeticException caught: " + e.getMessage());
} finally {
System.out.println("This block is always executed.");
}
}
}
Best Practices for Using Java Exceptions
-
Catch the Specific Exceptions: Always catch specific exceptions instead of using a generic
Exception
class. -
Clean-up Resources in
finally
Block: Use thefinally
block to clean up resources like close files or database connections. -
Avoid Using Exceptions for Control Flow: Exceptions should not be used as part of the regular program flow.
-
Document the Exceptions: Proper documentation helps future developers understand the potential errors.
Learning Resources
To enhance your Java skills, you may also want to delve into related topics, such as exploring the ‘this’ keyword in JavaScript or understanding Java arrays. You might also find it useful to learn techniques in other programming languages, like selecting options in JavaScript with Capybara from this JavaScript option Capybara tutorial or exploring various JavaScript coding tips.
By employing these exception handling techniques and learning from related programming paradigms, you can write more robust, efficient, and error-free code. Happy coding!